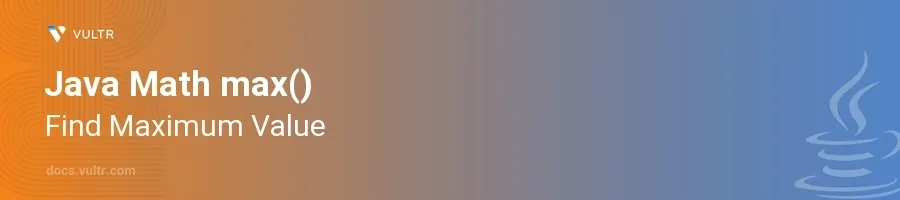
Introduction
The Math.max()
method in Java is a built-in function that is used to determine the maximum of two numbers. This method simplifies the task of comparing two numbers and returning the larger one, which is a common operation in many programming scenarios, such as statistical analysis, game development, or any application where finding the highest value is necessary.
In this article, you will learn how to effectively utilize the Math.max()
method in Java. Explore how to apply this method with different types of numbers including integers, floating-point numbers, and how to handle scenarios involving special values like NaN
(Not a Number).
Using Math.max() with Integers
Compare Two Integer Values
Define two integer variables.
Use the
Math.max()
method to determine which variable holds the larger value.javaint a = 5; int b = 10; int maximum = Math.max(a, b); System.out.println("The maximum value is: " + maximum);
This code compares
a
andb
, and sinceb
is greater thana
,Math.max()
returns the value ofb
.
Using Math.max() with Floating-Point Numbers
Compare Two Floating-Point Values
Define two floating-point variables.
Use the
Math.max()
method to find the maximum value.javadouble x = 5.5; double y = 3.3; double maximum = Math.max(x, y); System.out.println("The maximum value is: " + maximum);
In this snippet,
Math.max()
evaluatesx
andy
, and returnsx
because it holds the higher value.
Handle Special Cases
Consider scenarios that involve special floating-point values like
NaN
.Apply
Math.max()
to see how it behaves withNaN
.javadouble n1 = Double.NaN; double n2 = 1.0; double result = Math.max(n1, n2); System.out.println("Result with NaN: " + result);
Note that
Math.max()
returnsn2
when one of the values isNaN
. This is becauseNaN
is treated as less than any valid floating-point number, including negative infinity.
Using Math.max() in Practical Applications
Determine the Maximum of User Inputs
Use
Scanner
to take input from the user.Apply
Math.max()
to determine the largest input.javaScanner scanner = new Scanner(System.in); System.out.print("Enter first number: "); int firstNum = scanner.nextInt(); System.out.print("Enter second number: "); int secondNum = scanner.nextInt(); int result = Math.max(firstNum, secondNum); System.out.println("The greater number is: " + result); scanner.close();
This example collects two numbers from the user and compares them using
Math.max()
.
Conclusion
The Math.max()
function in Java offers a straightforward way to find the maximum value between two numbers. Ideal for applications across a broad spectrum of domains, this method enhances code efficiency and readability by abstracting away the need for manual comparisons. Implement Math.max()
in different scenarios, ranging from basic arithmetic operations to complex algorithms that require comparisons, to enforce optimal and clean code practices in your Java applications.
No comments yet.