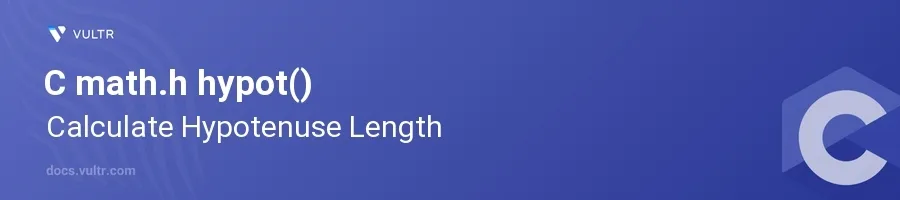
Introduction
The hypot()
function, available in C's math library math.h
, is specifically designed to calculate the hypotenuse of a right triangle given the lengths of the other two sides. This function is essential for accurately determining distances in various engineering and scientific calculations, adhering to the Pythagorean theorem.
In this article, you will learn how to effectively utilize the hypot()
function. Explore the precision and reliability of this function in calculating the length of the hypotenuse, even when working with very large or small numbers.
Basic Usage of hypot()
Calculate a Simple Hypotenuse
Include the math library in your program.
Declare variables for the two triangle sides.
Use
hypot()
to calculate the hypotenuse and output the result.c#include <stdio.h> #include <math.h> int main() { double side1 = 3.0; double side2 = 4.0; double hypotenuse = hypot(side1, side2); printf("Hypotenuse: %.2f\n", hypotenuse); return 0; }
In this code, the hypotenuse of a triangle with sides 3.0 and 4.0 is calculated using
hypot()
. The function returns5.00
, which is the expected result according to the Pythagorean theorem.
Handling Large Numbers
Recognize that
hypot()
manages large values well, reducing the risk of overflow errors common in manual calculations.Perform calculations with larger numbers.
c#include <stdio.h> #include <math.h> int main() { double side1 = 1e154; // A very large number double side2 = 1e154; // Another very large number double hypotenuse = hypot(side1, side2); printf("Hypotenuse of large numbers: %e\n", hypotenuse); return 0; }
This snippet demonstrates
hypot()
's effectiveness in handling and accurately computing the hypotenuse for extremely large numbers, where manually squaring such values would cause an overflow.
Using hypot() with Negative Values
Understand that
hypot()
always returns a non-negative value, suitable for geometric calculations.Apply the function to operands which include negative values.
c#include <stdio.h> #include <math.h> int main() { double side1 = -3.0; double side2 = 4.0; double hypotenuse = hypot(side1, side2); printf("Hypotenuse with negative side: %.2f\n", hypotenuse); return 0; }
This example confirms that
hypot()
handles negative inputs gracefully by treating them as positive distances, thereby returning the correct length of5.00
.
Conclusion
The hypot()
function is a dependable and precise tool in the C programming language for calculating the length of the hypotenuse in right-angled triangles. Its ability to handle large and negative values without error enhances its utility in complex mathematical computations and in fields requiring precise distance measurements. By incorporating hypot()
in your projects, streamline geometric calculations and ensure accuracy even under challenging scenarios. Remember to always link against the math library by using the -lm
flag when compiling your programs with gcc to avoid any linker errors.
No comments yet.