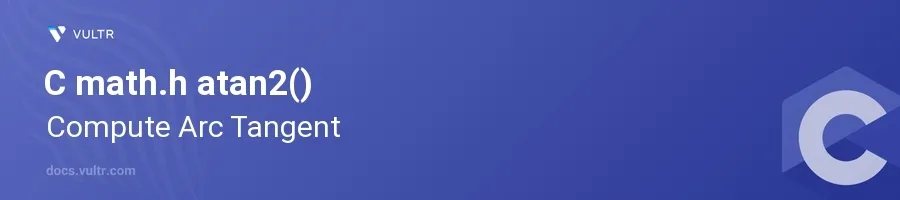
Introduction
The atan2()
function, part of C's math library in math.h
, computes the arc tangent of two variables, particularly useful for converting rectangular coordinates (x, y) to polar coordinates (r, \theta). This function returns the angle ( \theta ) between the ray ending at the origin and passing through the point ( (x, y) ), and the positive x-axis. The result is expressed in radians, and can handle all quadrants correctly due to the sign-aware inputs.
In this article, you will learn how to harness the atan2()
function in practical coding scenarios. Explore the essential steps to incorporate this function properly and understand the outcomes for different inputs, ensuring your ability to handle coordinate transformations and related calculations effectively.
Utilizing atan2() in C
Computing Angles with Cartesian Coordinates
Include the
math.h
header for accessing theatan2()
function.Create variables for Cartesian coordinates (x) and (y).
Call the
atan2()
function and print the result.c#include <stdio.h> #include <math.h> int main() { double x = 3.0; double y = 4.0; double theta = atan2(y, x); printf("Theta (radians): %f\n", theta); return 0; }
This code snippet calculates the angle ( \theta ) in radians for the point (3,0, 4,0) relative to the positive x-axis. The function
atan2(y, x)
efficiently determines the angle considering the correct quadrant.
Dealing with Various Quadrants
Prepare different
(x, y)
pairs for each quadrant.Apply the
atan2()
function for each pair.Print the computed angle for each point to understand how
atan2()
interprets different quadrants.c#include <stdio.h> #include <math.h> int main() { double coordinates[4][2] = {{1.0, 2.0}, {-1.0, 2.0}, {-1.0, -2.0}, {1.0, -2.0}}; for (int i = 0; i < 4; i++) { double x = coordinates[i][0]; double y = coordinates[i][1]; double theta = atan2(y, x); printf("Theta (radians) for (%f, %f): %f\n", x, y, theta); } return 0; }
In this code,
atan2()
calculates the angle for points located in all four quadrants. The output shows how the angle adjusts depending on the quadrant, ensuring accuracy in polar coordinates transformation.
Conclusion
Understanding and applying the atan2()
function from the C math library allows for precise computation of angles from Cartesian coordinates, critical for tasks such as converting coordinate systems or measuring angles. With this function, ensure that all quadrants are correctly interpreted and utilize these computations to simplify complex mathematical tasks. Mastering atan2()
enriches your toolkit as a C programmer, readying you for a wide range of scientific and analytical applications.
No comments yet.