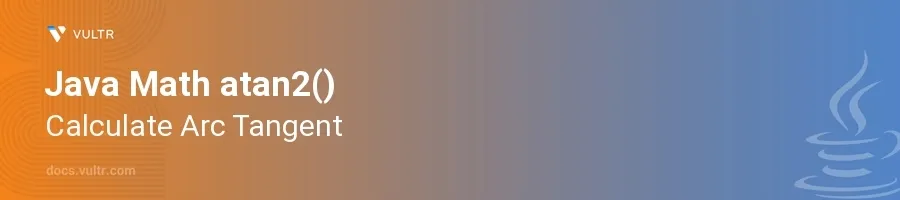
Introduction
The atan2()
method in Java's Math class is a useful function for calculating the angle theta from the conversion of rectangular coordinates (x, y) to polar coordinates (r, theta). This method returns the angle in radians between the positive x-axis of a plane and the point given by the coordinates (x, y). Often used in graphics, engineering, and wherever polar coordinates are involved, its capability extends from simple angle calculations to complex trigonometric operations.
In this article, you will learn how to utilize the atan2()
method in various scenario. Expanding upon typical use cases, you will see how atan2()
serves both basic and advanced mathematical calculations, helping developers and engineers to handle rotational dynamics efficiently.
Understanding the atan2() Method
Basic Usage of atan2()
Understand the signature and return type:
The method signature is
Math.atan2(double y, double x)
, and it specifically returns the angle in radians from the x-axis to a point (y, x). The angle returned is in the range -π to π.Calculate a simple angle:
To find the angle between the positive x-axis and the point (4, 4), use the following code:
javadouble y = 4; double x = 4; double angle = Math.atan2(y, x); System.out.println("The angle in radians is: " + angle);
Here
atan2()
calculates the angle, takingy
(vertical movement) andx
(horizontal movement) as inputs. The result is the angle in radians, indicating the direction of the point (4, 4) relative to the x-axis.
Dealing with Different Quadrants
Recognize that
atan2()
handles all four quadrants:The
atan2()
function automatically adjusts for the quadrant of the point, making it invaluable for full 360-degree directional calculations without needing quadrant checks.Explore an example with points in different quadrants:
javadouble[] yValues = {4, -4, -4, 4}; double[] xValues = {4, 4, -4, -4}; for (int i = 0; i < yValues.length; i++) { double angle = Math.atan2(yValues[i], xValues[i]); System.out.println("The angle in radians for quadrant " + (i + 1) + " is: " + angle); }
Each iteration calculates and prints the angle for a point in each of the four quadrants, demonstrating how
atan2()
adapts the result to the specific quadrant.
Advanced Use Cases of atan2()
Calculating Rotation Angles in Navigation
Utilize
atan2()
for dynamic angle calculations, such as in navigation where direction relative to a reference point is constantly changing:javadouble destinationY = 10; double destinationX = 10; double currentY = 6; double currentX = 3; double angleToTarget = Math.atan2(destinationY - currentY, destinationX - currentX); System.out.println("The navigation angle in radians is: " + angleToTarget);
In navigation, knowing the angle toward a target from your current position is crucial. Here,
atan2()
helps calculate that angle, making path adjustments clear and actionable.
Conclusion
The atan2()
function in Java's Math class offers a robust solution for calculating angles in radians between the x-axis and a point in any of the four quadrants. Its comprehensive handling of different coordinate signs and its straightforward output ranging from -π to π make it essential for scenarios requiring precise angular computation. From basic angle calculations to advanced applications in navigation and robotics, atan2()
provides both versatility and precision, enhancing mathematical processing in Java applications. Embrace these techniques to enhance the sophistication and accuracy of your mathematical computations in Java.
No comments yet.