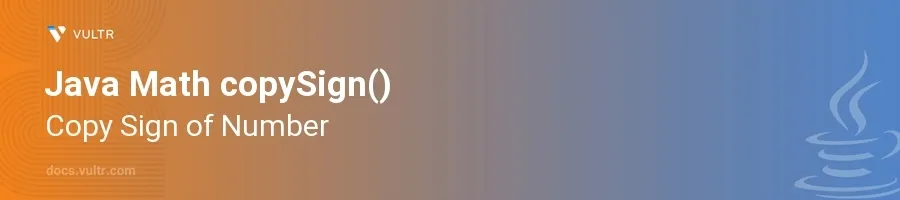
Introduction
The Math.copySign()
function in Java is a handy utility for manipulating the signs of floating-point numbers. This method copies the sign of one number to another, essentially delivering a target number with the desired sign, determined by the sign of another input. This capability is especially useful in complex mathematical simulations and calculations where control over the sign of a number can lead to more intuitive and readable code.
In this article, you will learn how to effectively employ the Math.copySign()
method in Java. Explore different scenarios where altering the sign of a number enhances the functionality of financial calculations, physics engines, or any computation requiring precision in sign manipulation.
Understanding Math.copySign()
Math.copySign()
is a method from the Java Math
class, designed to return the first floating-point argument with the sign of the second floating-point argument. It is particularly useful when maintaining the magnitude of the first argument but requiring the sign of the second.
Basic Usage of Math.copySign()
Define two numbers, where one serves as the source of the magnitude and the other as the source of the sign.
Use
Math.copySign()
to copy the sign from the second number to the first.javadouble magnitude = 3.5; double signSource = -2.0; double result = Math.copySign(magnitude, signSource); System.out.println(result);
This code will output
-3.5
since it copies the negative sign from-2.0
to3.5
.
Handling Special Cases
Consider scenarios involving special floating-point rules, such as handling
NaN
(Not a Number) and infinity.Apply
Math.copySign()
to examine its behavior in these edge cases.javadouble notANumber = Double.NaN; double negativeInfinity = Double.NEGATIVE_INFINITY; double resultNaN = Math.copySign(1.0, notANumber); double resultInfinity = Math.copySign(1.0, negativeInfinity); System.out.println("Result with NaN: " + resultNaN); System.out.println("Result with negative infinity: " + resultInfinity);
This example reveals that
Math.copySign()
handlesNaN
and negative infinity gracefully, returning the magnitude with the appropriate signs, maintaining the integrity of floating-point operations.
Practical Application
Utilize
Math.copySign()
within a financial application where adjusting the sign of transactions might be necessary.Implement a simple transaction adjustment mechanism.
javadouble transactionAmount = 950.50; double currentBalance = -200.00; // Negative balance implies debt // Ensure the sign of transaction matches the sign of the current balance (if in debt, transaction should reduce debt) double adjustedTransaction = Math.copySign(transactionAmount, currentBalance); System.out.println("Adjusted Transaction: " + adjustedTransaction);
Here,
Math.copySign()
ensures that the transaction helps reduce the debt by keeping the transaction as a negative value, enhancing the readability and accuracy of financial logs.
Conclusion
Master the Math.copySign()
function in Java to precisely control the sign of floating-point numbers across various computational contexts. This method exhibits flexibility and precision, particularly useful in scientific calculations, financial models, and any domain requiring explicit sign control. By leveraging Math.copySign()
, you gain the ability to guide numeric operations with greater confidence and clarity, ultimately leading to more robust and intuitive codebases.
No comments yet.