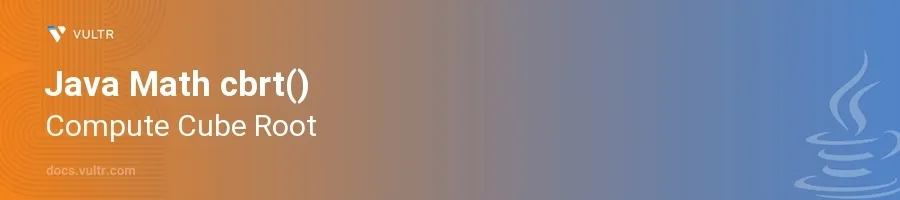
Introduction
In Java, the Math.cbrt()
method is a straightforward and efficient way to calculate the cube root of a number. This function is part of the Math
class in Java's standard library and is crucial for mathematical operations involving cube roots, prevalent in scientific calculations, geometry, physics, and 3D modeling.
In this article, you will learn how to use the Math.cbrt()
function in Java to compute the cube root of both positive and negative numbers. Follow through various examples that showcase the implementation and behavior of this method across different scenarios and data types.
Using Math.cbrt() in Java
Compute Cube Root of Positive Numbers
Start with a positive number whose cube root you wish to determine.
Utilize the
Math.cbrt()
method by passing the number as an argument.javadouble result = Math.cbrt(27); System.out.println("Cube root of 27 is: " + result);
This example calculates the cube root of 27. Since 3 cubed is 27,
Math.cbrt(27)
returns3.0
.
Compute Cube Root of Negative Numbers
Take a negative number for which the cube root needs to be calculated.
Apply
Math.cbrt()
similarly by enclosing the negative value.javadouble negativeResult = Math.cbrt(-8); System.out.println("Cube root of -8 is: " + negativeResult);
In this case, the cube root of
-8
is calculated. Because the cube of-2
is-8
,Math.cbrt(-8)
returns-2.0
.
Dealing with Non-Integer and Zero Values
Consider non-integer and zero values when using
Math.cbrt()
.Test the method on these diverse inputs to understand its behavior.
javadouble fromZero = Math.cbrt(0); double nonInteger = Math.cbrt(15.587); System.out.println("Cube root of 0: " + fromZero); System.out.println("Cube root of 15.587 is: " + nonInteger);
Here,
Math.cbrt(0)
outputs0.0
since the cube root of zero is zero. Moreover,Math.cbrt(15.587)
provides the cube root of a non-integer, demonstrating the method's capacity to process floating-point numbers accurately.
Conclusion
The Math.cbrt()
function in Java is a powerful component for computing the cube roots of numbers, including both positive and negative values. Due to its ease of use and versatility in handling different data types, it is highly beneficial for various applications requiring such mathematical calculations. Implement these examples to efficiently handle tasks requiring cube root calculations, ensuring your Java applications function with mathematical precision and clarity.
No comments yet.