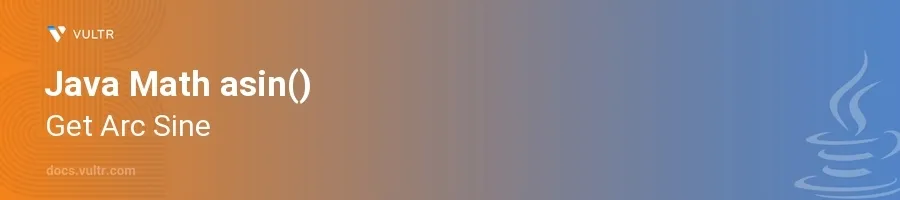
Introduction
The Math.asin()
method in Java is used to calculate the arc sine of a value, which is the inverse of the sine function. This function returns the angle in radians whose sine is the specified double value. It’s particularly useful in various fields such as trigonometry, physics, and engineering where angle measurements are necessary.
In this article, you will learn how to apply the Math.asin()
method to compute the arc sine of different values. Explore practical examples to understand how to apply this method correctly and how it behaves with various inputs.
Calculating Arc Sine using Math.asin()
Basic Use of Math.asin()
Define a double value within the range -1 to 1, as
Math.asin()
is defined for these values.Apply
Math.asin()
to calculate the arc sine of the value.javadouble value = 0.5; double result = Math.asin(value); System.out.println("Arc sine of " + value + " is: " + result + " radians");
This code calculates the arc sine of
0.5
. The result is returned in radians.
Edge Cases
Explore what happens when you use values at the boundary of the defined range.
javadouble minVal = -1; double maxVal = 1; System.out.println("Arc sine of -1: " + Math.asin(minVal) + " radians"); System.out.println("Arc sine of 1: " + Math.asin(maxVal) + " radians");
This snippet demonstrates the calculation of arc sine for the extreme values -1 and 1. These represent the minimum and maximum angles in the sine function range.
Values Beyond Valid Range
Examine the behavior of
Math.asin()
with values outside the valid range.javadouble outOfRangeValue = 1.5; double result = Math.asin(outOfRangeValue); System.out.println("Result: " + result);
When values outside of the -1 to 1 range are used, the method returns
NaN
, indicating the value is not a number because the input is outside the function's domain.
Conversion from Radians to Degrees
Convert the calculation result from radians to degrees to make it more intuitive, as degrees are often easier to interpret than radians.
javadouble value = 0.5; double radians = Math.asin(value); double degrees = Math.toDegrees(radians); System.out.println("Arc sine of " + value + " in degrees: " + degrees);
This code snippet converts the arc sine result from radians to degrees using
Math.toDegrees()
method, providing a result that might be more useful in certain contexts.
Conclusion
The Math.asin()
function in Java is an essential tool for calculating the arc sine of a number, offering a way to obtain angle measurements in radians for trigonometric applications. By understanding how to use this function and recognizing its limitations and behavior across different scenarios, enhance computational tasks related to angles efficiently. Use the techniques discussed to integrate arc sine calculations into Java applications effectively, ensuring accurate outcomes in trigonometric computations.
No comments yet.