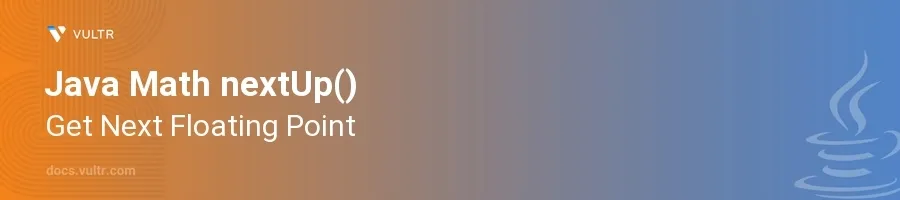
Introduction
The Math.nextUp()
method in Java is a precise tool used to find the next floating-point value that is slightly larger than a given number. This method is particularly useful in applications involving numerical computations where minute differences matter, such as in graphics rendering or scientific calculations where precision is crucial.
In this article, you will learn how to effectively use the Math.nextUp()
method in Java. Explore how this method works with both single and double precision floating point numbers, and see practical examples to understand its importance in ensuring numerical accuracy.
Understanding Math.nextUp()
Basics of Math.nextUp()
- Recognize that
Math.nextUp()
returns the smallest floating point value toward positive infinity from the argument. - Note that this method is part of the java.lang.Math package, which needs to be imported if not already.
Usage in Single Precision
Begin by defining a float value.
Apply
Math.nextUp()
to this float value.javafloat startingFloat = 0.1f; float nextFloat = Math.nextUp(startingFloat); System.out.println("Next up from " + startingFloat + " is " + nextFloat);
In this example,
Math.nextUp(startingFloat)
calculates the nearest greater floating point value to0.1f
. The result presents the smallest incrementation that moves the value towards positive infinity.
Usage in Double Precision
Start with a double value.
Use the
Math.nextUp()
method for this value.javadouble startingDouble = 1.0; double nextDouble = Math.nextUp(startingDouble); System.out.println("Next up from " + startingDouble + " is " + nextDouble);
Here, applying
Math.nextUp(startingDouble)
finds the smallest possible increment above1.0
. This instance showcases how precision affects floating-point calculations in double precision.
Practical Applications of Math.nextUp()
Ensuring Precision in Calculations
- Utilize
Math.nextUp()
in scenarios where computational accuracy is paramount, such as iterative algorithms and precision-sensitive calculations.
Avoiding Stagnation in Iterative Processes
- Use
Math.nextUp()
to guarantee that iteration values do not remain stagnant when expected to increment in finite precision environments. - Avoid issues where small differences can affect the outcome of algorithms, particularly in fields like computational geometry or scientific computing.
Conclusion
Java's Math.nextUp()
method is indispensable for achieving the highest accuracy in floating-point arithmetic. By learning how to use this function in both single and double precision contexts, ensure mathematical operations in Java are precise and reliable. Apply this method in various programming scenarios to handle edge cases where precision can significantly impact the results, fostering better stability and reliability in numerical applications.
No comments yet.