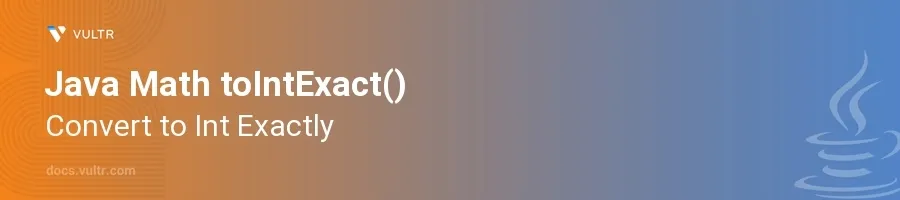
Introduction
The toIntExact()
method in Java is a crucial tool for safely converting long values to int when there is a guarantee that the value fits within the range of an int. This method helps in avoiding data loss due to narrowing primitive conversions between long and int types. It throws ArithmeticException
if the input value goes beyond the bounds of what can be represented as an int, ensuring reliability and data integrity in numerical computations.
In this article, you will learn how to leverage the toIntExact()
method in Java. Understand how to apply this function to ensure safe and precise data type conversions, with practical examples to demonstrate its usage in various programming scenarios.
Understanding the toIntExact() Method
Basic Usage of toIntExact()
Recognize the primary role of
toIntExact()
which is to convert a long value to an int safely.Import the Math class where
toIntExact()
is a static method.javalong value = 500L; int convertedValue = Math.toIntExact(value); System.out.println("The converted integer is: " + convertedValue);
Here, the long
value
is safely converted to an int without any risk of data loss because 500L is well within the range of an int.
Handling Out-of-Range Values
Be prepared for scenarios where the long value exceeds the int range.
Use
toIntExact()
with values that exceedInteger.MAX_VALUE
or are less thanInteger.MIN_VALUE
to see how it throwsArithmeticException
.javalong largeValue = 2147483648L; // This is 1 more than Integer.MAX_VALUE try { int result = Math.toIntExact(largeValue); } catch (ArithmeticException e) { System.out.println("Error: Value out of int range"); }
This code snippet properly captures the exception that arises from trying to convert a long value that doesn't fit within the int range.
Practical Use Case in a Real-world Application
Realize the importance of using
toIntExact()
in applications dealing with large numerical computations or data transport between systems with differing data sizes.Example scenario where large counter values could potentially be reduced: data aggregation processes where results might need to be narrowed down to integers for reporting or interfacing reasons.
javalong totalClicks = 123456789012L; try{ int displayClicks = Math.toIntExact(totalClicks); System.out.printf("Display on user interface: %d clicks", displayClicks); } catch (ArithmeticException e) { System.out.println("Total clicks exceeds display limit. Consider revising display metrics."); }
This example handles a potential overflow intelligently by catching the
ArithmeticException
, allowing the program to provide a meaningful message or alternative handling logic.
Conclusion
The toIntExact()
function in Java is a powerful method for ensuring type safety during conversions from long to int. Its built-in mechanism for exception handling makes it an essential choice for applications where precise control over numerical data is necessary. By integrating toIntExact()
in data processing routines, you ensure that your Java code adheres to strict data integrity and error handling standards, thus maintaining robustness and reliability in your applications. Use these techniques to safeguard your data conversions against common pitfalls associated with type narrowing.
No comments yet.