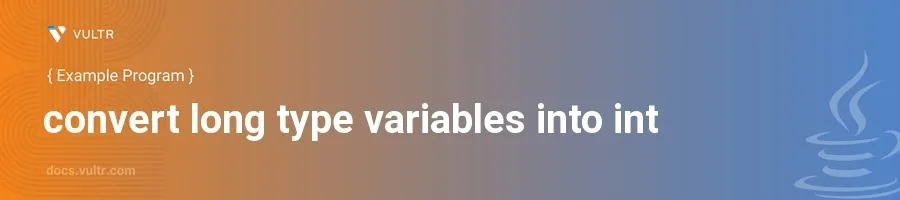
Introduction
The conversion of long type variables to int in Java is a common task that developers encounter. This typically involves casting the longer 64-bit long
data type into the shorter 32-bit int
type. Such transformations are necessary when interfacing with libraries or APIs that only accept integers, or when memory constraints and structures require smaller data types.
In this article, you will learn how to effectively convert long
variables into int
within a Java program using several practical examples. Grasp the nuances of type casting and understand the potential pitfalls such as data loss when the long
value exceeds the storage capacity of int
.
Basic Conversion Using Explicit Casting
Casting is the simplest method to convert a long
to an int
in Java. Here's how you can do it:
Declare a
long
variable.Cast the
long
to anint
using the explicit cast operator(int)
.javalong longValue = 1000L; int intValue = (int) longValue; System.out.println("Converted int value: " + intValue);
This code initializes a
long
variable and casts it toint
. Theprintln
statement then outputs the converted integer value.
Handling Larger Values
When dealing with long
values that exceed the range of int
, you must be cautious as casting can lead to data loss.
Example of Data Loss in Casting
Initialize a
long
variable with a value outside theint
range.Perform the cast and print the result.
javalong largeLongValue = 2147483648L; // Just above Integer.MAX_VALUE int resultInt = (int) largeLongValue; System.out.println("Unexpected int value: " + resultInt);
In this example, the
long
exceeds the maximum valueint
can hold, resulting in an overflow and incorrect result. TheSystem.out.println
confirms the unintended value.
Safely Managing Overflows
Check if the
long
value is within theint
range before casting.If not, handle the overflow appropriately by setting limits or giving alerts.
javalong longToConvert = 9223372036854775807L; // Long.MAX_VALUE int safeInt; if (longToConvert < Integer.MIN_VALUE || longToConvert > Integer.MAX_VALUE) { System.out.println("Warning: long value out of int range!"); safeInt = (int) Math.max(Integer.MIN_VALUE, Math.min(Integer.MAX_VALUE, longToConvert)); } else { safeInt = (int) longToConvert; } System.out.println("Safely converted int value: " + safeInt);
Here, the code checks the
long
value's bounds and prevents an overflow error by limiting the value to be within the acceptableint
range. It prints a warning if the value was out of range.
Advanced Usage: Converting Without Overflow
In some situations, you might want to ensure that a value wraps within the int
range correctly without simply truncating to maximum or minimum values.
Using Wraparound with Modulus
Take advantage of the modulus operator to wrap values within the
int
range.Demonstrate its application with a sample value.
javalong largeLong = 3000000000L; int wrappedInt = (int) (largeLong % 2147483648L); // Integer.MAX_VALUE + 1 System.out.println("Wrapped int value: " + wrappedInt);
This code uses the modulus to handle the wraparound, ensuring that the value stays within the integer limits without the need for range checks.
Conclusion
Converting long to int in Java requires careful handling to avoid data loss and overflow issues. By utilizing explicit casting and safeguarding against overflows, you maintain the integrity of your data and prevent exceptions or unexpected behavior in the application. From simply casting when you are sure the values are safe, to complex conditional logic for larger numbers, these techniques equip you to tackle type conversion challenges effectively in Java development scenarios. Apply these methods judiciously to maintain clean and robust code.
No comments yet.