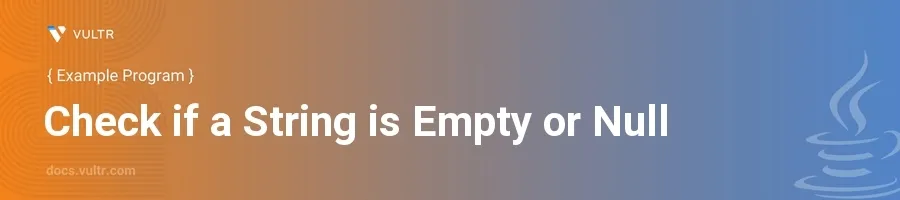
Introduction
In Java, checking whether a string is empty or null is a common operation, especially when dealing with user inputs or processing data where a string's presence needs to be validated. The Java language provides various ways to handle this, ensuring robustness and preventing runtime errors like NullPointerException
from derailing your application.
In this article, you will learn how to effectively check if a string is empty or null using several methods provided by Java. Explore practical examples that showcase these techniques to help you integrate them into your Java applications seamlessly.
Basic Empty and Null Check
Check Using Standard If-Else
Use the basic logical operations directly to check if a string is null or empty.
javapublic static boolean isNullOrEmpty(String str) { if (str == null || str.isEmpty()) { return true; } return false; }
This code checks if the variable
str
isnull
or empty (""
). If either condition is true, it returnstrue
; otherwise, it returnsfalse
.
Alternative Using Objects Class
Utilize the
Objects
utility class for a null-safe check.javapublic static boolean isNullOrEmpty(String str) { return str == null || str.isEmpty(); }
Similar to the previous example, this function returns
true
ifstr
isnull
or its length is zero. Using this one line enhances readability and reduces code complexity.
Leveraging Apache Commons Library
Using StringUtils.isEmpty()
Understand that the Apache Commons Lang library provides enhanced string handling utilities.
Add the Apache Commons Lang dependency to your project.
For Maven users, include this in your
pom.xml
:xml<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.12.0</version> </dependency>
Use
StringUtils.isEmpty()
to check for null or empty strings.javaimport org.apache.commons.lang3.StringUtils; public static boolean isNullOrEmpty(String str) { return StringUtils.isEmpty(str); }
StringUtils.isEmpty()
internally checks fornull
and empty strings, making your code cleaner and more expressive.
Using StringUtils.isBlank()
Understand the difference:
isBlank()
checks for null, empty, and whitespace only strings.Implement similar to
isEmpty()
but withisBlank()
for a broader check.javaimport org.apache.commons.lang3.StringUtils; public static boolean isNullOrEmpty(String str) { return StringUtils.isBlank(str); }
Here,
StringUtils.isBlank()
checks ifstr
is null, empty, or made solely of whitespace (spaces, tabs, new line characters). Very useful when the mere presence of whitespace should also classify the string as "empty".
Java 11 and Beyond: Using String Methods
Using String.isBlank() in Java 11
Recognize the enhancements in Java 11 including new String methods.
javapublic static boolean isNullOrEmpty(String str) { return str == null || str.isBlank(); }
With Java 11,
String.isBlank()
does whatStringUtils.isBlank()
from Apache Commons does but without the need for an external library.
Conclusion
Checking if a string is empty or null in Java can be approached in multiple ways, each serving different needs depending on your project requirements. From simple conditional checks and utilizing built-in Java methods to employing third-party libraries for more nuanced checks, choose the right method that fits your context. These methods enhance robustness, prevent common errors, and improve the overall safety and readability of your Java code. Implement these techniques in different scenarios to maintain high-quality code standards in your Java projects.
No comments yet.