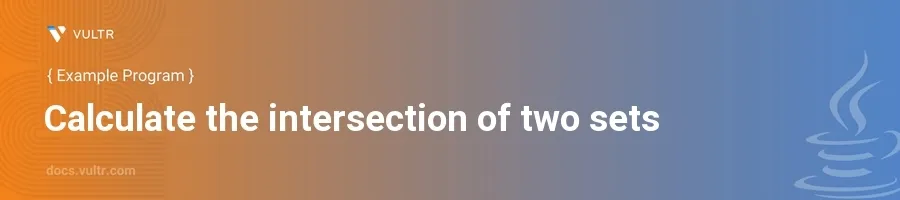
Introduction
In Java, calculating the intersection of two sets involves finding the common elements between them. This operation is fundamental in many applications like data analysis, where comparing datasets is frequent. Java Collections Framework provides straightforward methods to perform this, making the task efficient and less error-prone.
In this article, you will learn how to calculate the intersection of two sets in Java through practical examples. Explore various methods, including the use of standard library utilities and stream API features, to handle this operation effectively.
Utilizing Set Interface Methods
Basic Intersection with retainAll()
Create two
Set
objects filled with elements.Use the
retainAll()
method on one of the sets to retain only the elements that are present in both sets.javaSet<Integer> set1 = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5)); Set<Integer> set2 = new HashSet<>(Arrays.asList(3, 4, 5, 6, 7)); set1.retainAll(set2); System.out.println(set1);
The above code initializes two sets and uses
retainAll()
onset1
. This method modifiesset1
to keep only the elements that are also inset2
. The output will be[3, 4, 5]
, which are the common elements.
Intersection Without Modifying Original Sets
Initialize two
Set
objects with your preferred elements.Create a new set to store the intersection.
Use the
addAll()
method to copy elements from one set to the new set.Apply
retainAll()
to keep only the elements present in the second set.javaSet<Integer> originalSet1 = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5)); Set<Integer> originalSet2 = new HashSet<>(Arrays.asList(3, 4, 5, 6, 7)); Set<Integer> intersection = new HashSet<>(originalSet1); intersection.retainAll(originalSet2); System.out.println(intersection);
This snippet avoids altering the original sets by using a temporary set
intersection
. After copyingoriginalSet1
and applyingretainAll()
withoriginalSet2
,intersection
contains[3, 4, 5]
.
Leveraging Java Stream API
Stream-based Intersection
Recognize that Java's Stream API can be utilized for set operations in a functional style.
Convert one set to a stream and filter it by checking if each element exists in the other set.
Collect the results back into a set.
javaSet<Integer> setA = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5)); Set<Integer> setB = new HashSet<>(Arrays.asList(3, 4, 5, 6, 7)); Set<Integer> resultSet = setA.stream() .filter(setB::contains) .collect(Collectors.toSet()); System.out.println(resultSet);
In this example, the
filter()
method processes elements fromsetA
, retaining only those that are members ofsetB
. The result is collected intoresultSet
, which holds the intersection[3, 4, 5]
.
Conclusion
Calculating the intersection of two sets in Java can be executed efficiently using either traditional set operations provided by the Set
interface or through the expressive and fluid Stream API. Each method has its uses depending on the need to modify the original sets or the preference for a more functional programming approach. Practice these techniques to enhance your ability to handle sets in Java, making your code more efficient and your data processing tasks easier. By mastering these methods, you ensure your applications can handle complex data manipulations with ease.
No comments yet.