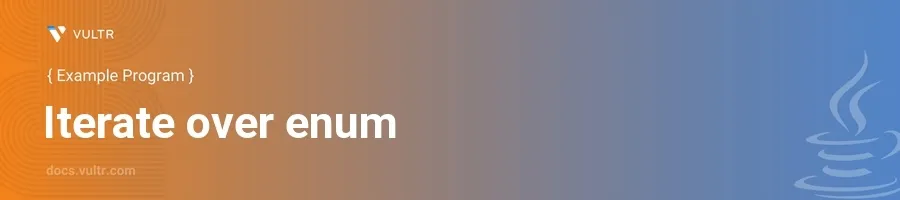
Introduction
Iterating over enums in Java is a common task performed in many Java applications, especially those involving state machines or specific pre-defined categories. Enums, or enumerated types, offer a way to define collections of constants in a clean and efficient manner. Exploring methods to iterate over these sets of constants can help improve the readability of your code and facilitate the handling of group-related data.
In this article, you will learn how to iterate over enums in various ways using Java. The methods discussed will include basic for-loops, enhanced for-loops, and using streams introduced in Java 8. Each method will be explained with its use cases, providing a comprehensive understanding of how these approaches can be integrated into your Java programs.
Basic Loop through an Enum
In Java, iterating directly over the values of an enum can be accomplished by leveraging the values()
method that every enum implicitly possesses. This method returns an array of the enum's constants, which can then be easily cycled through using a for-loop. Here's how you can implement this:
Example: Displaying All Enum Constants
Define an enum with a few constants.
Use a simple for-loop to iterate over these constants.
javapublic enum Season { SPRING, SUMMER, AUTUMN, WINTER } public class EnumIteration { public static void main(String[] args) { for (Season s : Season.values()) { System.out.println(s); } } }
This code defines an enum for the different seasons and then uses an enhanced for-loop to cycle through and print each season. When the
main
method executes, it outputs the names of each season as defined in the enum.
Using Java Streams to Iterate Over Enums
With the introduction of Streams in Java 8, iterating over elements including enums has become more functional and expressive. Streams allow for a high-level, functional-style operations on streams of elements, such as those provided by collections or arrays.
Example: Applying Functional Operations on Enum Constants
Use the
stream()
method to convert the enum constants to a stream.Apply stream operations to perform actions on the enum elements.
javaimport java.util.stream.Stream; public class EnumStreamIteration { public static void main(String[] args) { Stream.of(Season.values()) .forEach(System.out::println); } }
In this example, the
Stream.of()
method creates a stream from the array ofSeason
enum constants. TheforEach
method is then used to execute a print operation for each element in the stream, displaying each season.
Conditional Iteration Based on Attributes
Sometimes, the iteration might need to be conditional based on some attributes of the enums. Enums in Java can also have fields, methods, and constructors, making them extremely versatile.
Example: Filtering Enums Based on Custom Attributes
Expand the enum to include an attribute and a suitable method.
Use the stream to filter and process only relevant enum constants.
javapublic enum Season { SPRING(1), SUMMER(2), AUTUMN(3), WINTER(4); private int quarter; Season(int quarter) { this.quarter = quarter; } public int getQuarter() { return quarter; } } public class EnumAttributeIteration { public static void main(String[] args) { Stream.of(Season.values()) .filter(s -> s.getQuarter() % 2 == 0) .forEach(s -> System.out.println(s + " occurs in quarter " + s.getQuarter())); } }
In this code, each
Season
enum constant is associated with a quarter. The stream is filtered to include only those seasons that occur in even-numbered quarters. Consequently, it only prints out the seasons that meet the specified condition.
Conclusion
Iterating over enums in Java provides a structured approach to manage fixed sets of related constants. By utilizing loops, streams, and conditional logic, you can efficiently traverse through these constants and incorporate necessary logic based on application requirements. Whether using simple for-loops to handle basic iterations or streams for more expressive and complex filtering, Java's support for enums ensures you have the tools necessary to manipulate and utilize these special data types effectively in your programs. Experiment with the examples provided and harness the power of enums in your next Java project.
No comments yet.