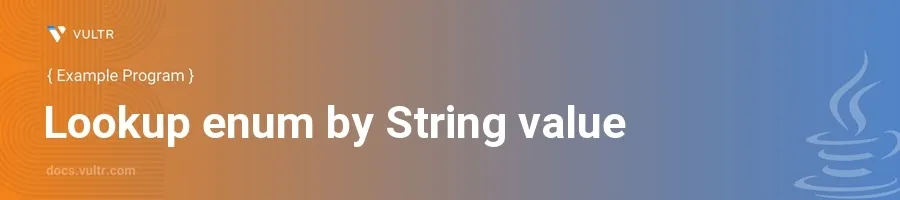
Introduction
Enums in Java are types that have a fixed set of constants, making them incredibly useful for creating collections of constant values such as directions, days of the week, and more complex settings like command-line flags or state codes. Often, there's the need to look up an enum by a String value, for instance when parsing configuration files or command-line arguments where the inputs are in a textual format.
In this article, you will learn how to effectively use Java Enums to handle scenarios where a String value needs to be converted into a defined Enum type. You will explore different methods of achieving this with practical code examples, which will guide you through the process of creating enums, and implementing lookup methods using both switch statements and HashMaps.
Understanding Enums in Java
Basic Enum Creation
Define a simple Enum for demonstration. For example, creating an enum called
Day
that represents days of the week.javapublic enum Day { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY }
This code snippet defines a
Day
enum with values for each day of the week.
Lookup with values() and valueOf()
Understand that every enum in Java inherently has a static
values()
method, which returns an array of all enum constants in the order they're declared.Consider
valueOf()
method which is a powerful method provided by Java enums. It takes a string and returns the corresponding enum constant.javaDay day = Day.valueOf("MONDAY"); System.out.println(day);
The
valueOf()
method throws anIllegalArgumentException
if the specified name doesn't match any of the enum constants.
Strategies for Enum Lookup by String
Using a Switch Statement
Implement a method that utilizes a switch statement to convert a string to its corresponding
Day
enum constant.javapublic static Day getDayByString(String dayStr) { switch (dayStr.toUpperCase()) { case "MONDAY": return Day.MONDAY; case "TUESDAY": return Day.TUESDAY; case "WEDNESDAY": return Day.WEDNESDAY; case "THURSDAY": return Day.THURSDAY; case "FRIDAY": return Day.FRIDAY; case "SATURDAY": return Day.SATURDAY; case "SUNDAY": return Day.SUNDAY; default: throw new IllegalArgumentException("Invalid Day: " + dayStr); } }
This method converts a given string to uppercase and uses a
switch
on the result to determine which enum constant to return.
Using a HashMap for Lookup
Realize the benefit of using a
HashMap
to store string-to-enum mappings for quick lookups.Create a static HashMap to map strings to
Day
enums.javaimport java.util.HashMap; import java.util.Map; public enum Day { // Enum constants MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY; // HashMap to hold string to enum mapping private static final Map<String, Day> lookup = new HashMap<>(); static { for (Day day : Day.values()) { lookup.put(day.name(), day); } } public static Day get(String day) { return lookup.get(day.toUpperCase()); } }
This initializes a
HashMap
whenDay
is loaded, mapping each day's name to its enum constant. The static methodget()
can then be used to perform fast lookups by string.
Conclusion
Implementing a lookup system for enums by String values in Java is a common requirement in applications needing to parse external data into structured formats. Both switch
statements and HashMap
methodologies provide robust solutions to this problem. Decide which approach suits the project at hand, taking into consideration the simplicity of switch statements against the speed and scalability of HashMap. With these techniques mastered, efficiently handle and convert string inputs to their respective enums in Java, enhancing data handling capabilities of your applications.
No comments yet.