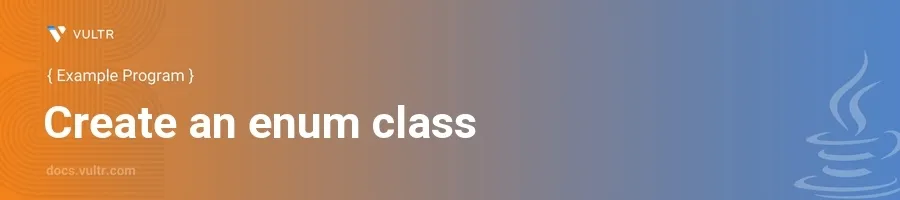
Introduction
In Java, an enum
(enumeration) is a special Java type used to define collections of constants. More robust than a simple list of constants, an enum can include methods, variables, and constructors. They are often used when you need a fixed set of constants, such as days of the week, states in a process, levels of an organization, etc., making your code more maintainable and readable.
In this article, you will learn how to define and utilize enum
classes in Java through practical examples. Explore how to declare an enum, handle its constants, and how to integrate enums effectively in your Java programs.
Defining a Simple Enum in Java
Basic Enum Declaration
Start by declaring a basic enum with a few constants. Here, consider an example of an enum representing the days of the week.
javapublic enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
This code snippet defines an
enum
calledDay
with seven constants, representing each day of the week. Each constant in an enum is an instance of the enum class.
Accessing Enum Constants
Access these constants via the enum name followed by a dot (
.
), and the constant name.javaDay today = Day.MONDAY; System.out.println("Today is " + today);
This snippet declares a variable
today
of typeDay
and initializes it withDay.MONDAY
. It then prints the current day.
Enum with Attributes and Methods
Adding Attributes and Constructors to Enum
Enhance your enum by adding attributes and a constructor. This additional complexity allows for more detailed state retention and methods inside your enums.
javapublic enum Color { RED("#FF0000"), GREEN("#00FF00"), BLUE("#0000FF"); private final String code; Color(String code) { this.code = code; } public String getCode() { return code; } }
Here, the
Color
enum includes a private fieldcode
, which stores the hexadecimal code for each color. The constructorColor(String code)
allows for initializing these fields when the constant is created.
Using Enum Methods
Utilize the method defined in the enum to perform operations.
javaColor favoriteColor = Color.BLUE; System.out.println("Favorite Color Code: " + favoriteColor.getCode());
This code sets
favoriteColor
toColor.BLUE
and uses thegetCode()
method to retrieve and print the hexadecimal value of the color.
Enum in Switch Statements
Utilizing Enum in Control Structures
Leverage enums in a switch statement to make decisions based on enum values.
javaDay day = Day.TUESDAY; switch(day) { case MONDAY: System.out.println("Start of the work week."); break; case FRIDAY: System.out.println("Almost weekend!"); break; default: System.out.println("Midweek days."); break; }
This snippet demonstrates using an enum in a switch statement to execute different blocks of code depending on the enum value. Such usage enhances readability and the maintainability of conditional logic.
Conclusion
Through defining and using enums in Java, you augment the readability and robustness of your code. Enums offer a structured way to handle fixed sets of constants with optional associated functionalities. Whether you’re managing simple constants like days of the week, or more complex data such as color codes with methods, enums make your Java programs easier to manage and maintain. Harness these strategies in your own development to elevate the clarity and effectiveness of your code.
No comments yet.