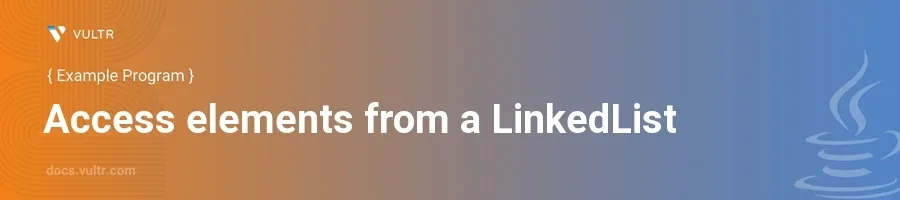
Introduction
LinkedList in Java is a part of the Java Collections Framework and it is used extensively when performance in insertion or deletion operations is critical. It provides a doubly linked list implementation of the List and Deque interfaces, allowing for efficient positional access and alteration of elements.
In this article, you will learn how to access elements from a Java LinkedList. Discover the various methods provided by the LinkedList class to retrieve or check elements. Each section of this article guides you through different examples and explains the results.
Accessing Elements in LinkedList
Retrieve the First and Last Element
Create an instance of a
LinkedList
.Add some elements to the list.
Use the
getFirst()
andgetLast()
methods to retrieve the first and last elements respectively.javaLinkedList<String> list = new LinkedList<>(); list.add("Apple"); list.add("Banana"); list.add("Cherry"); String firstElement = list.getFirst(); String lastElement = list.getLast(); System.out.println("First Element: " + firstElement); System.out.println("Last Element: " + lastElement);
The output of the code would be:
First Element: Apple Last Element: Cherry
getFirst()
fetches the first element ('Apple') andgetLast()
fetches the last element ('Cherry') from the LinkedList.
Accessing Elements by Index
Understand that
LinkedList
allows accessing elements by their index, similar to an array or anArrayList
.Use the
get(int index)
method to retrieve an element at a specific position in the LinkedList.javaString elementAtIndexTwo = list.get(2); System.out.println("Element at Index 2: " + elementAtIndexTwo);
This code fetches the element at index 2, which is 'Cherry'.
Check if an Element Exists
Sometimes, it's necessary to check whether a LinkedList contains a particular item before proceeding.
Use the
contains(Object o)
method to check if an element is present in the list.javaboolean containsBanana = list.contains("Banana"); System.out.println("Contains Banana: " + containsBanana);
If 'Banana' is an element in the list, the method returns
true
.
Iterating Through LinkedList
To access all elements in a
LinkedList
, iterating through the list is commonly used.Utilize
for
loops or enhancedfor
loops to iterate over elements.javafor (String item : list) { System.out.println(item); }
This loop prints all elements: 'Apple', 'Banana', and 'Cherry'.
Using Iterators
LinkedList implementations support various iterator types including basic, descending, and ListIterator.
Use iterators to traverse the LinkedList in different orders.
javaIterator<String> iterator = list.iterator(); while (iterator.hasNext()) { System.out.println("Item via Iterator: " + iterator.next()); } Iterator<String> descendingIterator = list.descendingIterator(); while (descendingIterator.hasNext()) { System.out.println("Item via Descending Iterator: " + descendingIterator.next()); }
The first snippet uses a regular iterator to access elements in the order they were added, while the second uses a descending iterator to access elements in reverse order.
Conclusion
Effectively accessing elements from a LinkedList in Java includes using methods like getFirst()
, getLast()
, and get(index)
, or by employing iterators for more complex traversal needs. Whether accessing a single element or iterating through the LinkedList, Java provides multiple methods to suit different requirements. Through the examples provided, embrace these techniques to enhance manipulation and retrieval operations in LinkedLists, ensuring efficient and effective usage in your Java applications.
No comments yet.