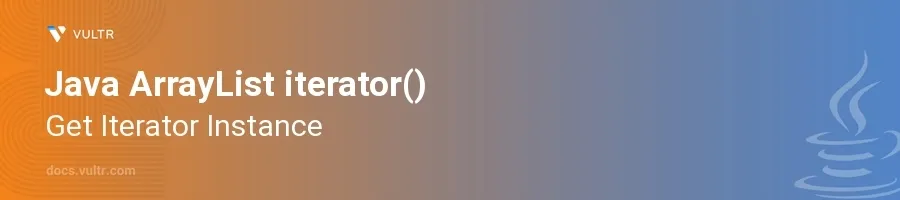
Introduction
In Java, the ArrayList
is a part of the Java Collections Framework, extensively used for its dynamic array capabilities and flexibility. The ability to iterate over the elements of an ArrayList
seamlessly is crucial in many programming scenarios, especially when you need to traverse or modify elements.
In this article, you will learn how to use the iterator()
method of the ArrayList
class to get an Iterator instance. This will enable you to traverse the list in a forward direction, inspect elements, and make modifications during iteration using safe operations provided by the Iterator interface.
The iterator() Method from ArrayList
The iterator()
method is a powerful way to gain sequential access to elements within an ArrayList
. Here’s how to effectively use this method:
Obtain an Iterator from an ArrayList
Initialize an
ArrayList
.Use the
iterator()
method to obtain anIterator
.javaimport java.util.ArrayList; import java.util.Iterator; ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Cherry"); Iterator<String> it = fruits.iterator();
In this code, an
ArrayList
of strings is created, and elements are added to it. Theiterator()
method is then called to retrieve anIterator
over the elements of theArrayList
.
Using the Iterator to Traverse the ArrayList
Check if there are more elements using
hasNext()
.Access the next element using
next()
.javawhile (it.hasNext()) { String fruit = it.next(); System.out.println(fruit); }
This loop will iterate through each element in the
ArrayList
until all elements have been accessed. ThehasNext()
method checks if there’s a next element, and thenext()
method retrieves it.
Modifying the List During Iteration
Use the
remove()
method of the Iterator to safely remove elements while iterating.javawhile (it.hasNext()) { String fruit = it.next(); if (fruit.equals("Banana")) { it.remove(); } } System.out.println(fruits);
This iteration will remove "Banana" from the
ArrayList
. Theremove()
method provided by the Iterator ensures that the underlying collection is modified safely during the iteration process.
Conclusion
Using the iterator()
method in conjunction with an ArrayList in Java provides a robust way to traverse and manipulate elements in a collection. This method ensures safe modifications during iteration, preventing common errors such as ConcurrentModificationException
. By mastering the use of the Iterator instance, you enable more dynamic and error-free handling of elements within lists, enhancing both the functionality and reliability of your Java applications.
No comments yet.