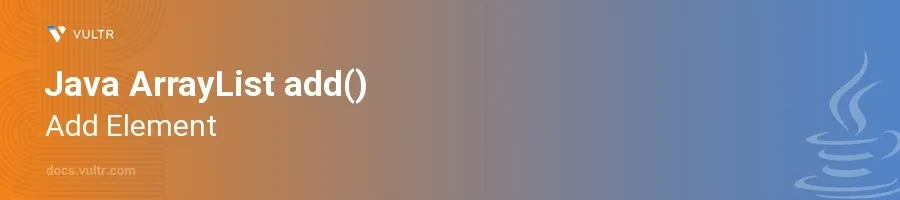
Introduction
The add()
method in Java's ArrayList
class is one of the most frequently used operations for dynamically managing collections of objects. This method allows for the insertion of elements into an ArrayList, providing flexibility and ease of use in modifying list contents during runtime.
In this article, you will learn how to add elements to an ArrayList
using the add()
method. Explore how to append elements, insert them at specific positions, and understand how the method impacts the ArrayList's underlying structure.
Adding Elements to ArrayList
Appending Elements to an ArrayList
Ensure you have an instance of an
ArrayList
.Use the
add()
method to append an element at the end of the list.javaimport java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); System.out.println("Fruits List: " + fruits); } }
In this code, two elements, "Apple" and "Banana," are appended to the
fruits
ArrayList. Theadd()
method adds these elements to the end of the list.
Inserting Elements at a Specific Position
Make sure the
ArrayList
already exists.Use
add(int index, E element)
to insert an element at a desired index.javaimport java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add(1, "Cherry"); System.out.println("Fruits List: " + fruits); } }
This example first adds "Apple" and "Banana" to the list, then inserts "Cherry" at index 1. The original "Banana" element shifts to the next index, demonstrating how elements after the insertion point are moved to accommodate the new element.
Managing ArrayList Capacity
Understanding Automatic Resizing
ArrayList
in Java automatically increases its capacity when you add elements and it reaches its current capacity.- This resizing is managed internally, but understanding it helps in optimizing performance especially with large lists.
Adding elements to an ArrayList
is a simple yet powerful operation that supports both straightforward appends and precise positional insertions. Through the use of the add()
method, Java provides flexible and dynamic array management that is crucial for many applications dealing with collections of data.
Conclusion
The add()
method of the Java ArrayList
class is essential for dynamically adjusting the contents of lists during runtime. By adding elements either at the end of the list or at specific indices, you accommodate diverse programming needs. As you integrate this functionality into Java applications, you benefit from the flexibility and performance optimizations inherent to ArrayList
. Whether dealing with user inputs, processing data, or managing internal states, utilizing the add()
method ensures that your lists remain both accessible and efficient.
No comments yet.