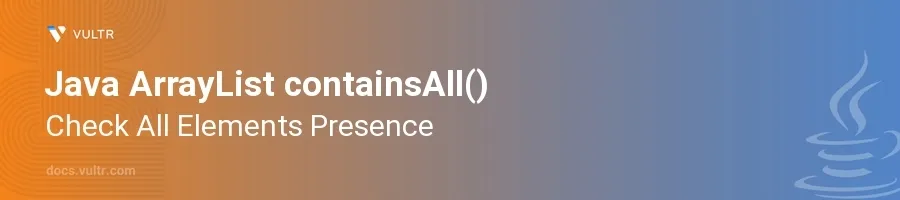
Introduction
The containsAll()
method in Java's ArrayList
class is designed to determine if one list contains all elements of another list. This method is particularly useful when you need to verify if a subset of elements is entirely present within a larger collection, making it a common utility in collection manipulation and verification tasks.
In this article, you will learn how to utilize the containsAll()
method effectively. Explore its application through practical examples and understand how to integrate it into Java programs to check for the presence of all elements from one list within another.
Understanding containsAll() Method
Basic Usage of containsAll()
Create the main
ArrayList
and a sublist whose presence you want to verify.Use the
containsAll()
method to check if all elements of the sublist are present in the main list.javaimport java.util.ArrayList; import java.util.Arrays; ArrayList<Integer> mainList = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5)); ArrayList<Integer> subList = new ArrayList<>(Arrays.asList(2, 3)); boolean result = mainList.containsAll(subList); System.out.println("All elements found: " + result);
This code initializes
mainList
with elements andsubList
with a subset of the same elements. UsingcontainsAll()
, it checks whether all elements ofsubList
are present inmainList
. The output will betrue
since elements 2 and 3 exist in themainList
.
Handling Different Data Types
Understand that
containsAll()
can be used withArrayLists
of any data type.Create
ArrayLists
of a non-integer type, such asString
, and perform the check.javaArrayList<String> mainList = new ArrayList<>(Arrays.asList("apple", "banana", "cherry")); ArrayList<String> subList = new ArrayList<>(Arrays.asList("banana", "cherry")); boolean result = mainList.containsAll(subList); System.out.println("All elements found: " + result);
Here, both
mainList
andsubList
contain strings. The method confirms that both "banana" and "cherry" fromsubList
are found inmainList
, resulting in atrue
output.
Practical Applications of containsAll()
Data Filtering
Use
containsAll()
to filter data based on multiple criteria.Suppose a scenario where you filter records based on the presence of specified elements.
javaArrayList<String> data = new ArrayList<>(Arrays.asList("red", "blue", "green", "yellow")); ArrayList<String> filterCriteria = new ArrayList<>(Arrays.asList("blue", "green")); boolean isDataValid = data.containsAll(filterCriteria); System.out.println("Data meets criteria: " + isDataValid);
In this example,
data
represents a dataset andfilterCriteria
represents the necessary conditions. The outputtrue
indicates that all required criteria are present in the data.
Validity Checks
Implement
containsAll()
for integrity checks, ensuring all required fields or items are present in a collection.Use a checklist scenario where all necessary items must be checked off.
javaArrayList<String> checklist = new ArrayList<>(Arrays.asList("passport", "tickets", "visa")); ArrayList<String> itemsPacked = new ArrayList<>(Arrays.asList("passport", "visa", "tickets", "sunglasses")); boolean isReadyToTravel = itemsPacked.containsAll(checklist); System.out.println("Ready to travel: " + isReadyToTravel);
This example uses
containsAll()
to verify that all necessary travel items (passport, tickets, visa) are packed. The method returnstrue
, indicating readiness for travel.
Conclusion
The containsAll()
method in Java's ArrayList
class is a versatile tool for verifying the presence of all elements from one collection within another. By integrating this function into your Java applications, you enhance your ability to perform comprehensive checks and data validations efficiently. Whether for simple presence checks or complex data integrity validations, understanding how to use containsAll()
effectively empowers you to handle collections more proficiently.
No comments yet.