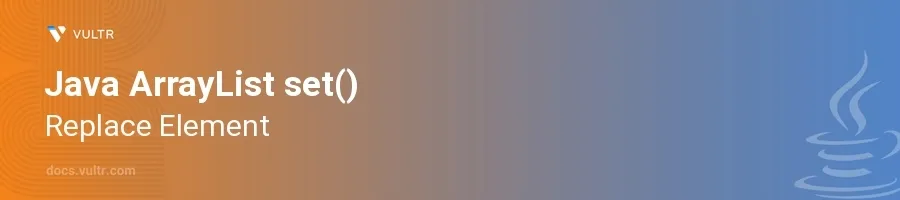
Introduction
The set()
method in Java's ArrayList
class is a crucial tool for modifying elements in a list. It allows the replacement of an element at a specified position, facilitating dynamic data management within your Java applications. This method ensures that lists can be updated efficiently without the need to create new lists or perform cumbersome operations.
In this article, you will learn how to utilize the ArrayList
set()
method to replace elements. Explore practical examples that demonstrate replacing elements in single and multi-dimensional ArrayLists and understand how this method interacts with Java's collection framework.
Understanding the set() Method
Basic Usage of set()
Ensure you have an
ArrayList
created and populated with initial values.Determine the index at which you want to replace an element.
Use the
set()
method to replace the element at the specified index.javaArrayList<String> colors = new ArrayList<>(Arrays.asList("Red", "Green", "Blue")); colors.set(1, "Yellow"); // Replace "Green" with "Yellow" System.out.println(colors);
This code initializes an
ArrayList
of colors, replaces the second element "Green" with "Yellow", and prints the updated list.
Error Handling
Be aware that using an invalid index will throw an
IndexOutOfBoundsException
.Always check if the index is within the bounds of the list size before attempting to set a value.
javaArrayList<String> colors = new ArrayList<>(Arrays.asList("Red", "Green", "Blue")); if (1 < colors.size()) { colors.set(1, "Yellow"); System.out.println(colors); } else { System.out.println("Index out of bounds"); }
Here, the code checks if the index is valid before trying to replace the element. This prevents the program from crashing due to an index out of bounds exception.
Advanced Usage in Multi-dimensional ArrayList
Replacing Elements in a 2D ArrayList
Understand that a 2D ArrayList is essentially an ArrayList of ArrayLists.
Access the inner ArrayList using the outer index, then use
set()
on the inner ArrayList.javaArrayList<ArrayList<Integer>> matrix = new ArrayList<>(); matrix.add(new ArrayList<>(Arrays.asList(1, 2, 3))); matrix.add(new ArrayList<>(Arrays.asList(4, 5, 6))); matrix.add(new ArrayList<>(Arrays.asList(7, 8, 9))); matrix.get(0).set(1, 20); // Replace 2 with 20 in the first row System.out.println(matrix);
In this example, the
set()
method is used to replace the number 2 with 20 in the first innerArrayList
. The alteration reflects in the entire matrix structure when printed.
Conclusion
The set()
method in Java's ArrayList
provides a simple yet powerful way to manipulate list data. By replacing elements directly within the list, you maintain the efficiency of your data structures and ensure your applications can adapt to changing data requirements. With the understanding gained from these examples, leverage the set()
method in both simple and complex data structures to optimize your Java applications' performance and reliability.
No comments yet.