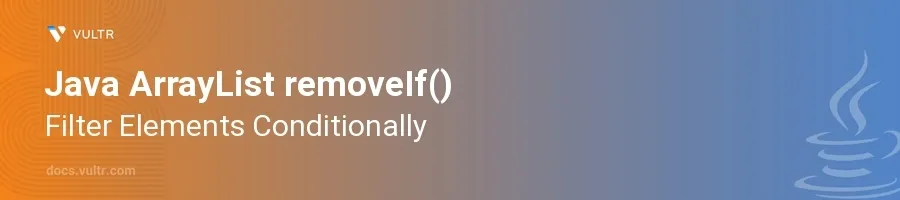
Introduction
The removeIf()
method in Java's ArrayList
class offers a straightforward way to remove elements from a list based on a condition specified via a lambda expression or predicate. This functionality becomes immensely useful when you need to filter out elements without manually iterating through the entire list, providing both cleaner code and improved performance for certain operations.
In this article, you will learn how to effectively use the removeIf()
method in various practical scenarios. The discussion covers how to define conditions for element removal, integrate more complex predicates, and utilize this method in real-world data manipulation tasks.
Understanding the removeIf() Method
Basic Usage of removeIf()
Start with a simple
ArrayList
of elements.Apply
removeIf()
with a straightforward condition.javaArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9)); numbers.removeIf(n -> n % 2 == 0); System.out.println(numbers);
This code effectively removes all even numbers from the
numbers
list. The conditionn -> n % 2 == 0
is a lambda expression that evaluates totrue
for even numbers.
Complex Conditions
Define an
ArrayList
with more complex types, such as custom objects or collections of data.Use a compound predicate in the
removeIf()
method.javaArrayList<String> strings = new ArrayList<>(Arrays.asList("Hello", "World", "Java", "Code", "removeIf")); strings.removeIf(s -> s.length() > 4 && Character.isLowerCase(s.charAt(0))); System.out.println(strings);
In this example, strings that are longer than 4 characters and start with a lowercase letter are removed. The condition combines length checking and character evaluation.
Using removeIf() with Method Reference
Consider using method references to further simplify the code especially when calling existing methods that return a boolean.
Create another scenario where method references improve readability.
javaArrayList<String> phrases = new ArrayList<>(Arrays.asList("apple", "banana", "cherry", "date")); phrases.removeIf(String::isEmpty); System.out.println(phrases);
Here,
String::isEmpty
is a method reference that is passed toremoveIf()
, removing any empty strings from thephrases
list.
Real-World Applications
Cleaning Data
Imagine handling a list of user-generated content where you need to remove null values or trim empty spaces and then remove empty entries.
Utilize
removeIf()
in a chained approach for efficient data cleaning.javaArrayList<String> comments = new ArrayList<>(Arrays.asList(" Great post! ", null, "Thanks!", "", " Very informative! ")); comments.removeIf(Objects::isNull); comments.replaceAll(String::trim); // Trims all remaining entries comments.removeIf(String::isEmpty); System.out.println(comments);
This sequence first removes all
null
values, trims whitespace from the remaining strings, and then removes all the empty strings, tremendously simplifying data processing pipelines.
Conclusion
The removeIf()
function in Java's ArrayList
is a powerful mechanism for removing elements based on specific conditions, thus facilitating a more expressive and cleaner approach compared to traditional iteration methods. With the ability to integrate complex predicates and method references, this method enhances code readability and efficiency. Harness the removeIf()
method in various situations from simple data list cleanups to more complex data processing tasks to maintain clean and effective codebases.
No comments yet.