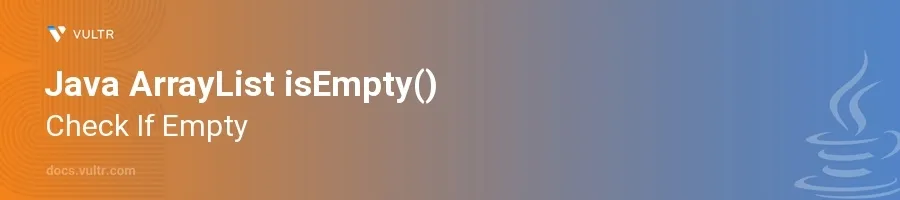
Introduction
The isEmpty()
method in Java's ArrayList
class is a straightforward tool to check if the list contains no elements. It returns true
if the list is empty; otherwise, it returns false
. This method provides a clear way to verify list contents before performing operations that require the list to have elements, such as accessing or deleting items, which can help to avoid errors such as IndexOutOfBoundsException
.
In this article, you will learn how to leverage the isEmpty()
method in various use cases involving ArrayLists
. Explore how this method can act as a safeguard in your code by preventing operations on empty lists, thus maintaining the robustness and efficiency of your Java applications.
Understanding isEmpty() Method
Basic Usage
Initialize an
ArrayList
.Use the
isEmpty()
method to check if the list is empty.javaArrayList<String> stringList = new ArrayList<>(); boolean isListEmpty = stringList.isEmpty(); System.out.println("Is the list empty? " + isListEmpty);
This code initializes an empty
ArrayList
ofString
type and checks if it is empty using theisEmpty()
method. It printstrue
because the list has no elements.
Checking Before Operations
Avoid performing operations on empty lists to prevent runtime errors.
Use
isEmpty()
to validate the list's state before accessing elements.javaArrayList<Integer> numbers = new ArrayList<>(); if(numbers.isEmpty()) { System.out.println("The list is empty, cannot perform operations."); } else { System.out.println("The first element is: " + numbers.get(0)); }
In this snippet, the
isEmpty()
method checks if thenumbers
list is empty. Since it’s true, the condition prevents attempting to access the first element, which would lead to an error due to the list being empty.
Integrating isEmpty() with Logical Conditions
Combine
isEmpty()
with other logical operations to handle multiple conditions effectively.Make decisions based on the list's status alongside other criteria.
javaArrayList<Double> prices = new ArrayList<>(); prices.add(19.99); prices.add(15.99); if (!prices.isEmpty() && prices.get(0) > 18) { System.out.println("The first price is higher than 18 and the list is not empty."); }
The code first checks if the
prices
list is not empty and then checks if the first item in the list is greater than 18. Both conditions must be true to execute the print statement. This method ensures that the operations inside theif
block are safe from exceptions related to accessing elements in an empty list.
Conclusion
Utilizing the ArrayList
isEmpty()
method in Java helps prevent errors by ensuring that list operations are carried out only when there are elements present. Whether checking if the list is empty before an operation, combining it with other conditions, or managing logical decisions based on list content, isEmpty()
enhances the safety and reliability of your Java code. Adapt and integrate this method to ensure robust list handling in your Java applications.
No comments yet.