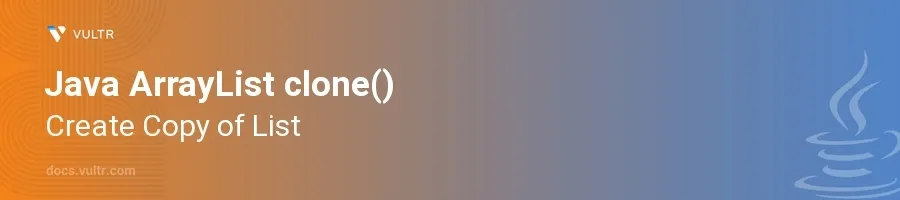
Introduction
The clone()
method in Java's ArrayList
class is a technique for creating a duplicate of the existing list with a shallow copy of its elements. This function can be crucial when you need to retain the original list untouched while manipulating the copied list. This method is particularly useful in scenarios where the original list should not be altered for the sake of data integrity and bug avoidance.
In this article, you will learn how to effectively use the clone()
method to duplicate an ArrayList
. This includes understanding the type of copy it creates, handling the returned object, and practical usage examples demonstrating its application.
Understanding the clone() Method
Concept of Shallow Copy
- Recognize that
clone()
performs a shallow copy of theArrayList
. This means it copies the references of the objects contained in the list, not the objects themselves. - If the list contains objects and you modify those objects, both the original and cloned lists will reflect these changes. However, any structural modifications to the list itself, such as adding or removing elements, will not affect the other list.
Syntax and Return Type
- The
clone()
method returns an object of typeObject
, thus requiring explicit casting toArrayList
. - Ensure safe usage by checking the type and possibly handling
ClassCastException
.
Sample Syntax
ArrayList original = new ArrayList();
ArrayList copy = (ArrayList) original.clone();
This code snippet demonstrates the basic usage of the clone()
method where original
is an ArrayList
that is being cloned into copy
. It's essential to cast the result of clone()
back to ArrayList
.
Practical Examples
Cloning a List of Strings
Create and populate an
ArrayList
of string type.Clone the list using
clone()
and cast the result.Display the original and cloned list.
javaArrayList<String> original = new ArrayList<>(); original.add("Java"); original.add("Python"); original.add("C++"); ArrayList<String> clonedList = (ArrayList<String>) original.clone(); System.out.println("Original List: " + original); System.out.println("Cloned List: " + clonedList);
This code creates a list of programming languages, clones it, and prints both lists. Changes to the content of the elements in the list (string manipulation) will not affect either list encase strings are immutable objects.
Cloning a List of Objects
Create a simple class to demonstrate cloning with mutable objects.
Populate an
ArrayList
with instances of this class.Clone the list and modify an object to show that both lists are affected.
javaclass Book { String title; public Book(String title) { this.title = title; } @Override public String toString() { return "Book{" + "title='" + title + '\'' + '}'; } } ArrayList<Book> books = new ArrayList<>(); books.add(new Book("Effective Java")); books.add(new Book("Java Concurrency")); ArrayList<Book> clonedBooks = (ArrayList<Book>) books.clone(); books.get(0).title = "Effective Java 3rd Edition"; System.out.println("Original List: " + books); System.out.println("Cloned List: " + clonedBooks);
In this scenario, modifying the title of a
Book
object in the original list also reflects in the cloned list due to the shallow copy nature ofclone()
.
Conclusion
The clone()
method of the ArrayList
class in Java is a handy tool to create a shallow copy of lists. Its application is simple yet requires careful handling to ensure that operations do not unintentionally affect both the original and cloned lists, especially when the lists contain mutable objects. By following the techniques and examples provided, you can effectively utilize this method to duplicate lists safely and efficiently.
No comments yet.