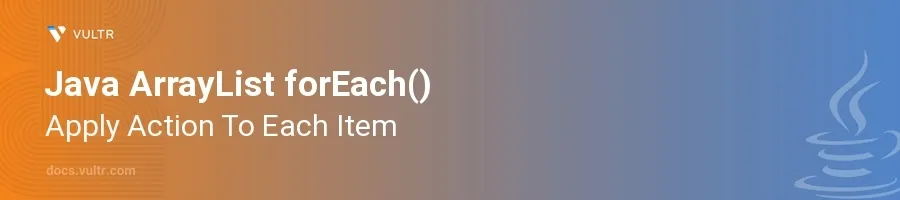
Introduction
The forEach()
method in Java's ArrayList
class represents a modern approach to iterating over elements, providing a streamlined mechanism to execute actions on each item. It leverages the capabilities of lambda expressions introduced in Java 8, simplifying the iteration process without explicitly handling iterators or for loops.
In this article, you will learn how to efficiently utilize the forEach()
method with ArrayLists in Java. Discover the scenarios where this method enhances code readability and maintainability, and explore practical examples demonstrating its use with lambda expressions and method references.
Utilizing forEach() with Lambda Expressions
Execute a Simple Action on Each Element
Prepare an ArrayList with sample elements.
Apply the
forEach()
method to print each element.javaList<String> items = Arrays.asList("Apple", "Banana", "Cherry"); items.forEach(item -> System.out.println(item));
This example prints each string element in the
items
list. The lambda expressionitem -> System.out.println(item)
is executed for each element.
Modify Elements
Work with an ArrayList of integers.
Use
forEach()
to perform operations that modify each element.javaList<Integer> numbers = Arrays.asList(1, 2, 3); numbers.forEach(number -> System.out.println(number * 2));
This code doubles each number in the
numbers
list and prints it. It illustrates how to useforEach()
to apply computational actions directly.
Leveraging Method References
Use System.out.println as a Method Reference
Initialize an ArrayList of elements.
Pass
System.out::println
to theforEach()
method to print elements.javaList<String> cities = Arrays.asList("New York", "London", "Tokyo"); cities.forEach(System.out::println);
Using
System.out::println
as a method reference simplifies the code by directly linking the method without specifying the input explicitly.
Invoke Custom Methods
Define a class with a method that performs an action on a member.
Use
forEach()
to call this method on each instance.javaclass Printer { public void print(String message) { System.out.println("Message: " + message); } } List<String> messages = Arrays.asList("Hello", "World", "Java"); Printer printer = new Printer(); messages.forEach(printer::print);
In this example, each string in
messages
is passed to theprint
method of thePrinter
class instance, highlighting how method references can link to instance methods.
Conclusion
The forEach()
method in the Java ArrayList class provides a robust and concise way to iterate over and manipulate elements with minimal code. Incorporating lambda expressions and method references empowers developers to write cleaner, more readable code. Leverage this method to enhance the efficiency and clarity of your Java collections handling. Through practical application, continue to explore and utilize forEach()
in various programming contexts to streamline your development process.
No comments yet.