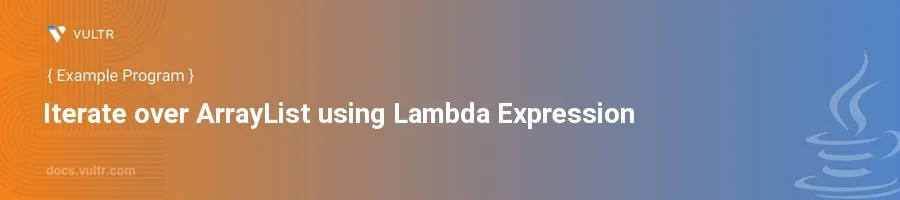
Introduction
The use of lambda expressions in Java simplifies the process of iterating over collections such as ArrayLists
. Introduced in Java 8, lambda expressions provide a clear and concise way to represent one-method interfaces using an expression. They are particularly useful for implementing functional programming concepts in Java, making tasks like iterating over collections, filtering data, and applying operations on each element much simpler.
In this article, you will learn how to effectively leverage lambda expressions to iterate over an ArrayList
in Java. Discover techniques and examples that enhance readability and efficiency in handling collections, ranging from simple print operations to more complex data manipulations.
Basic Iteration with Lambda Expressions
Using lambda expressions for iterating over ArrayList
elements introduces a more functional style of writing Java code. Here's how you can achieve this:
Print Elements of an ArrayList
First, create an
ArrayList
and add some elements to it.Use the
forEach
method with a lambda expression to print each element.javaimport java.util.ArrayList; ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Cherry"); fruits.forEach(item -> System.out.println(item));
In this code,
fruits.forEach(item -> System.out.println(item))
iterates over each element of thefruits
ArrayList and prints it. The lambda expressionitem -> System.out.println(item)
specifies the action to be performed on each element.
Modify Elements Before Printing
Start with an
ArrayList
ofInteger
.Use
forEach
to modify and print elements, such as printing their squares.javaimport java.util.ArrayList; ArrayList<Integer> numbers = new ArrayList<>(); numbers.add(1); numbers.add(2); numbers.add(3); numbers.forEach(number -> System.out.println(number * number));
Here, each number in
numbers
is squared and printed. The lambda expressionnumber -> System.out.println(number * number)
modifies each element before printing.
Using Lambda Expressions for Condition-Based Actions
Lambda expressions paired with conditional logic offer powerful solutions for custom iterations over ArrayList
.
Print Elements Based on Condition
Create an
ArrayList
with elements.Use
forEach
along with a conditional statement inside the lambda expression to selectively print elements.javaimport java.util.ArrayList; ArrayList<Integer> ages = new ArrayList<>(); ages.add(18); ages.add(22); ages.add(16); ages.forEach(age -> { if (age >= 18) { System.out.println(age + " is eligible."); } else { System.out.println(age + " is not eligible."); } });
In this example, each age is checked for eligibility (being 18 or older). The lambda expression includes a multi-line block, allowing for more complex operations like conditional logic.
Advanced Usage of Lambda in Collection Operations
Lambda expressions can not only iterate but also seamlessly integrate with other Java Stream API operations for more sophisticated manipulations.
Filter and Execute Actions
Prepare an
ArrayList
with mixed elements.Stream the elements, filter them, and perform an action on the filtered set.
javaimport java.util.ArrayList; ArrayList<String> names = new ArrayList<>(); names.add("John"); names.add("Sarah"); names.add("Sue"); names.stream() .filter(name -> name.startsWith("S")) .forEach(name -> System.out.println(name + " starts with S"));
This code uses
stream()
coupled withfilter()
andforEach()
. It filters out names that start with the letter "S" and then prints them. The lambda infilter()
defines the criteria, while the lambda inforEach()
defines the action.
Conclusion
Lambda expressions in Java provide a compact and expressive way to handle operations on collections, especially for iterating over ArrayList
. They not only reduce the boilerplate code but also enhance the readability and maintainability of your Java applications. Experimenting with lambda expressions in various scenarios like simple iterations, condition-based actions, and integrated stream operations unlocks extensive possibilities in functional programming in Java. By mastering these techniques, you ensure your Java code is both efficient and modern.
No comments yet.