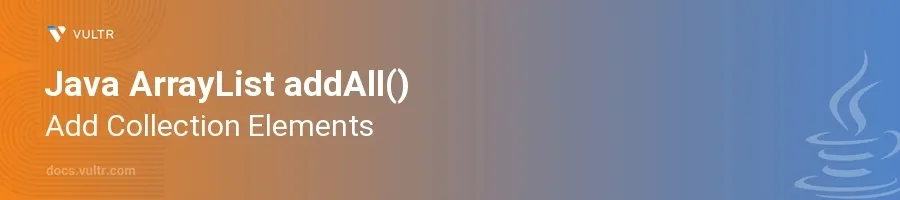
Introduction
The addAll()
method in Java's ArrayList
class is a critical tool for developers, providing a way to add all elements of a collection to an ArrayList. This method is especially useful when you need to merge data from multiple collections without manually iterating over each element. The ability to efficiently combine lists helps in creating more readable and maintainable code.
In this article, you will learn how to effectively use the addAll()
method in various scenarios. Discover how to merge collections seamlessly, handle duplicates, and increase the performance of your Java applications through practical examples.
Understanding addAll()
Basic Usage of addAll()
Instantiate an ArrayList and a second collection from which elements are to be added.
Use the
addAll()
method to merge the second collection into the ArrayList.javaArrayList<String> list1 = new ArrayList<>(); list1.add("Apple"); list1.add("Banana"); ArrayList<String> list2 = new ArrayList<>(); list2.add("Cherry"); list2.add("Date"); list1.addAll(list2); System.out.println(list1);
This example demonstrates merging
list2
intolist1
. The final output will be[Apple, Banana, Cherry, Date]
, showing that elements fromlist2
have been added tolist1
after its initial elements.
Checking Return Value
Realize that
addAll()
returns a boolean value.Use this return value to check if the list changed as a result of the call.
javaArrayList<Integer> nums1 = new ArrayList<>(); nums1.add(1); nums1.add(2); ArrayList<Integer> nums2 = new ArrayList<>(); nums2.add(3); nums2.add(4); boolean isChanged = nums1.addAll(nums2); System.out.println("List changed: " + isChanged); // Outputs true
In the snippet,
isChanged
captures the return value ofaddAll()
. Since elements are added,addAll()
returnstrue
.
Advanced Usage of addAll()
Adding Collections at Specific Index
Initiate an ArrayList and populate it with initial elements.
Use
addAll(int index, Collection<? extends E> c)
to add elements from another collection at a specific position.javaArrayList<String> colors = new ArrayList<>(); colors.add("Red"); colors.add("Blue"); ArrayList<String> moreColors = new ArrayList<>(); moreColors.add("Green"); moreColors.add("Yellow"); colors.addAll(1, moreColors); System.out.println(colors);
In this code,
moreColors
is inserted intocolors
starting at index 1. The resulting ArrayList will be[Red, Green, Yellow, Blue]
, illustrating how elements are added starting at the specified index.
Handling Duplicates
Understand that
addAll()
does not inherently manage duplicates.Decide on the logic to handle duplicates based on the application needs.
javaHashSet<String> set = new HashSet<>(); ArrayList<String> listWithDuplicates = new ArrayList<>(); listWithDuplicates.add("Apple"); listWithDuplicates.add("Apple"); // Duplicate value set.addAll(listWithDuplicates); System.out.println(set); // Outputs [Apple]
Here, a
HashSet
is used to ensure no duplicates are added, demonstrating how to handle duplicate elements when usingaddAll()
by employing a set that doesn’t allow duplicates.
Conclusion
The addAll()
function in Java's ArrayList
is indispensable for developers needing to merge collections efficiently. Its versatility extends from adding all elements at once to inserting collections at a specific position in the list. By mastering addAll()
, developers can ensure their Java applications run more smoothly and their code base remains clean and efficient. Utilize this method to enhance data handling in your Java projects, ensuring optimal performance and readability.
No comments yet.