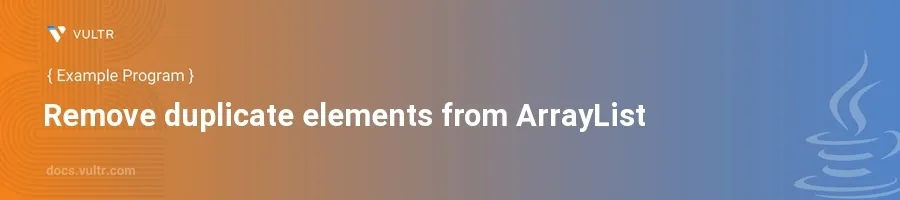
Introduction
Java's ArrayList
is a resizable array implementation of the List
interface. It's a part of the Java Collections Framework, commonly used for its dynamic array features that provide the flexibility to add and remove items. Handling duplicate elements in ArrayLists
is a common task, whether for data cleanup, ensuring data integrity, or simply meeting specific application requirements.
In this article, you will learn how to remove duplicate elements from an ArrayList
using different methods in Java. Understand how to implement these techniques to ensure your lists contain only unique elements, optimizing both space and processing time.
Using HashSet to Remove Duplicates
Convert ArrayList to HashSet
Import the necessary classes.
Create an
ArrayList
with duplicate elements.Initialize a
HashSet
using theArrayList
to eliminate duplicates.Convert the
HashSet
back to anArrayList
.javaimport java.util.ArrayList; import java.util.HashSet; import java.util.List; public class RemoveDuplicates { public static void main(String[] args) { List<Integer> listWithDuplicates = new ArrayList<>(); listWithDuplicates.add(1); listWithDuplicates.add(2); listWithDuplicates.add(2); listWithDuplicates.add(3); HashSet<Integer> hashSet = new HashSet<>(listWithDuplicates); List<Integer> listWithoutDuplicates = new ArrayList<>(hashSet); System.out.println("List without duplicates: " + listWithoutDuplicates); } }
This code effectively removes duplicates by leveraging the unique nature of
HashSet
. TheArrayList
is converted to aHashSet
which automatically drops all duplicate items since a set only allows unique elements. Subsequently, the unique values are transferred back to anArrayList
.
Using Java 8 Streams to Remove Duplicates
Stream Distinct Method
Utilize Java’s Stream API for a modern approach.
Stream the
ArrayList
, apply thedistinct()
method, and collect the result.javaimport java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; public class RemoveDuplicatesStream { public static void main(String[] args) { List<Integer> listWithDuplicates = new ArrayList<>(); listWithDuplicates.add(1); listWithDuplicates.add(2); listWithDuplicates.add(2); listWithDuplicates.add(3); List<Integer> listWithoutDuplicates = listWithDuplicates.stream() .distinct() .collect(Collectors.toList()); System.out.println("List without duplicates: " + listWithoutDuplicates); } }
This snippet uses the
stream()
method of theList
interface, which processes the elements in a functional approach. Thedistinct()
method filters out the duplicates, andcollect()
gathers the elements back into a list. Streams are especially useful for their code readability and functional programming capabilities in concurrent environments.
Conclusion
Removing duplicate elements from an ArrayList
in Java can be efficiently managed using either a HashSet
or the Java 8 Streams API. Each method offers different advantages: HashSet
provides a straightforward approach to eliminating duplicates, while streams offer a more expressive and robust option, particularly useful in a functional programming context. Choose the method that best fits your situation but ensure performance and readability are optimized in your Java applications. By applying the techniques discussed, manage your lists effectively and maintain data quality.
No comments yet.