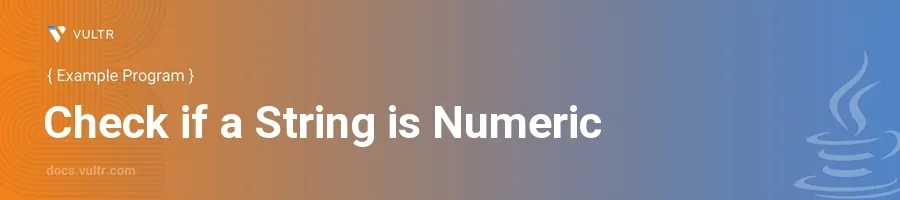
Introduction
Validating whether a string is numeric encompasses determining if it represents a number, including integers or decimals, without causing an exception during parsing. This validation is crucial in applications where user input needs to be strictly numerical, such as in monetary calculations or mathematical computations.
In this article, you will learn how to accurately validate if a string is numeric in Java using different examples. Dive into various methods, including the use of regular expressions, built-in Java functions, and try-catch blocks, which are necessary to handle potential parsing errors. These methodologies provide a comprehensive way to ensure that your program correctly identifies numeric strings in different formats.
Using Java Exception Handling
Try-Catch with Parsing
Use
Integer.parseInt()
orDouble.parseDouble()
method wrapped in a try-catch block.Check for
NumberFormatException
which occurs if the string cannot be parsed as an integer or double.javapublic static boolean isNumericUsingException(String str) { try { Double.parseDouble(str); return true; } catch (NumberFormatException e) { return false; } }
This function attempts to parse the string as a double. If successful, it returns
true
, otherwisefalse
if aNumberFormatException
is thrown, indicating that the string is not numeric.
Key Points to Consider
- Catch Specific Exception: It's good practice to catch the most specific exception (
NumberFormatException
here) rather than a general exception. - Handling Negative and Decimal Numbers: This method can correctly handle negative and decimal numbers because it uses
Double.parseDouble()
.
Using Regular Expressions
Pattern Matching
Define a regular expression that matches numeric strings which can include negatives and decimals.
Use
Pattern
andMatcher
classes fromjava.util.regex
package to evaluate the string.javapublic static boolean isNumericUsingRegex(String str) { return str.matches("-?\\d+(\\.\\d+)?"); }
This regex pattern:
-?
allows for an optional minus character for negatives.\\d+
ensures one or more digits exist.(\\.\\d+)?
optionally allows for a decimal followed by one or more digits.
Key Points to Consider
- Coverage: Regex can be tailored to match exactly the format you need (e.g., including scientific notation).
- Performance Considerations: While regex is powerful, it can be slower than parsing for larger strings or more complex patterns.
Using Apache Commons Validator
Using Library Functions
Add Apache Commons Validator library to your project, which features robust utility methods including numeric validation.
Utilize the
isDigits()
method which checks if a string is a positive integer, andisNumber()
for more generalized numeric formats including decimals and negatives.javapublic static boolean isNumericUsingApache(String str) { return org.apache.commons.validator.routines.NumberValidator.getInstance().isValid(str); }
This utilizes the
NumberValidator
to directly validate the string against various numeric formats.
Key Points to Consider
- Ease of Use: Using library functions can save time and makes your code cleaner.
- Dependency Management: Remember that adding external libraries increases the size of your project and may introduce version compatibility issues.
Conclusion
Checking if a string is numeric in Java allows you to avoid runtime errors and ensures that user inputs meet the expected format. From using standard Java try-catch blocks and parsing methods to employing regular expressions and leveraging third-party libraries like Apache Commons, choose the method that best matches your project’s complexity and performance needs. Employ these strategies to enhance data validation in your applications, ensuring safer and more efficient handling of numeral data inputs.
No comments yet.