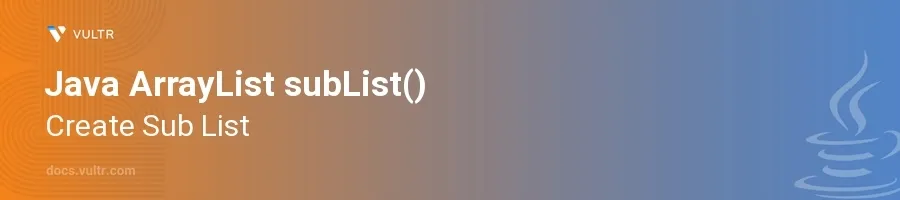
Introduction
The subList()
method in Java's ArrayList
class is a convenient way to create a new list that is a portion of an existing list. This method is particularly helpful for operations that require manipulating or analyzing subsets of data stored in an ArrayList. Typically used in data processing, pagination, and windowing algorithms, subList()
offers a flexible solution for handling list segments efficiently.
In this article, you will learn how to utilize the subList()
method across various practical scenarios. Explore how to create sublists, modify the original list's contents through sublists, and understand the implications these modifications have on the original list.
Using subList() in Java
Extracting Sublists
Start with a populated
ArrayList
of elements.Use the
subList()
method to create a sublist from the original list, specifying the start and end indices.javaArrayList<String> colors = new ArrayList<>(Arrays.asList("Red", "Orange", "Yellow", "Green", "Blue", "Indigo", "Violet")); List<String> warmColors = colors.subList(0, 3); System.out.println(warmColors);
This code snippet extracts the first three elements of the ArrayList
colors
, creating a sublist containing"Red"
,"Orange"
, and"Yellow"
. ThesubList()
method takes two parameters: the start index (inclusive) and the end index (exclusive).
Modifying the Original List via a Sublist
Understand that the sublist returned by
subList()
is backed by the original list.Make modifications to this sublist (such as adding or removing elements) and observe changes in the original ArrayList.
javawarmColors.add("Black"); System.out.println(colors);
By adding
"Black"
towarmColors
, the originalcolors
list is also updated. This behavior is crucial to understand as it demonstrates the "backed" nature of sublists. Any structural modification to a sublist affects the original list.
Handling Concurrent Modification Exception
Recognize that modifications to the sublist can sometimes lead to a
ConcurrentModificationException
if the original list is modified directly during operations on the sublist.Ensure exclusive access or handle modifications attentively to avoid such exceptions.
javacolors.add("Pink"); // Add element after subList creation try { System.out.println(warmColors); } catch (ConcurrentModificationException e) { System.out.println("List structure modified, cannot access sublist"); }
Adding
"Pink"
tocolors
after the sublistwarmColors
is created can lead to inconsistency and throw aConcurrentModificationException
when accessingwarmColors
. This example highlights the importance of managing modifications carefully when working with sublists and their parent lists.
Conclusion
Utilizing the subList()
function in Java's ArrayList
efficiently manipulates subsections of larger lists. Comprehend the implications of sublist modifications on the original list and manage concurrent modifications to avoid runtime exceptions. Whether it's for data slicing, batch processing, or any segment-based operations, the subList()
method enhances flexibility in handling lists, making your code more modular and easier to maintain. By mastering these techniques, ensure your list manipulations are both efficient and effective.
No comments yet.