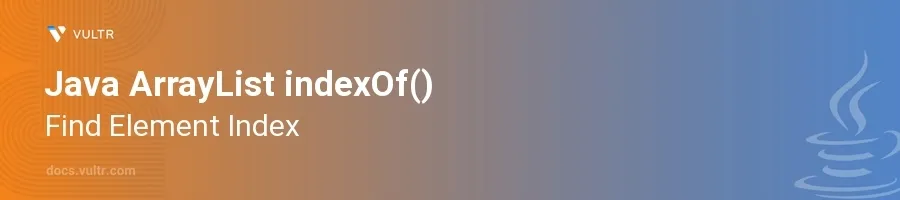
Introduction
The indexOf()
method in Java's ArrayList is crucial for determining the first occurrence index of a particular element. This method is particularly beneficial when you need to locate the position of an element to perform further operations like sorting, updating, or conditionally processing elements based on their positions.
In this article, you will learn how to effectively use the indexOf()
method in the ArrayList. Explore strategies to handle various scenarios including handling non-existent elements, using the method with custom object types, and optimizing search operations in larger lists.
Using indexOf() in ArrayList
Find the Index of a Simple Element
Create an instance of ArrayList and add some elements to it.
Use the
indexOf()
method to find the index of a specified element.javaimport java.util.ArrayList; ArrayList<String> list = new ArrayList<>(); list.add("Apple"); list.add("Banana"); list.add("Cherry"); int index = list.indexOf("Banana"); System.out.println("Index of 'Banana': " + index);
This code initializes an ArrayList with three string elements and uses
indexOf()
to find the index of "Banana". If found, it prints the index, otherwise, it returns-1
.
Handling Non-existent Elements
Understand that
indexOf()
returns-1
if the element is not found.Implement checks to handle this return value appropriately.
javaArrayList<Integer> numbers = new ArrayList<>(); numbers.add(1); numbers.add(2); numbers.add(3); int numIndex = numbers.indexOf(4); System.out.println("Index of number 4: " + (numIndex != -1 ? numIndex : "Not found"));
In this example, the number
4
is not in the list, soindexOf()
returns-1
. The code handles this by checking the return value and printing "Not found".
Using indexOf() with Custom Objects
Implement the
equals()
method in your custom class to ensureindexOf()
works correctly.Create a list of custom objects and use
indexOf()
to find the index of a specific object.javaimport java.util.ArrayList; class Fruit { String name; public Fruit(String name) { this.name = name; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; Fruit fruit = (Fruit) obj; return name.equals(fruit.name); } } ArrayList<Fruit> fruits = new ArrayList<>(); fruits.add(new Fruit("Apple")); fruits.add(new Fruit("Banana")); Fruit searchFruit = new Fruit("Banana"); int fruitIndex = fruits.indexOf(searchFruit); System.out.println("Index of 'Banana': " + fruitIndex);
This snippet defines a
Fruit
class with an overriddenequals()
method, ensuring thatindexOf()
correctly compares the objects based on thename
property.
Conclusion
The indexOf()
function in Java's ArrayList is a powerful tool for finding the index of elements within the list. Mastery of this function allows you to manipulate lists more efficiently, especially when combined with custom object types or when handling large datasets. By using the techniques discussed, you can enhance your Java applications, making them more efficient and robust when dealing with list-based data structures.
No comments yet.