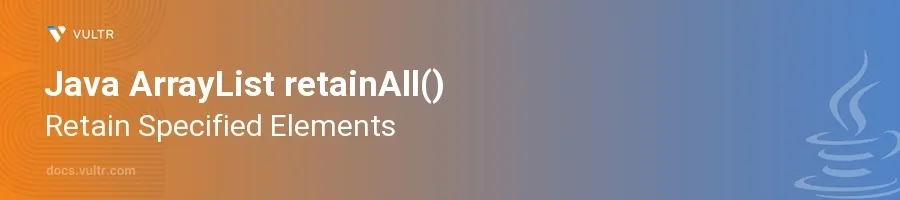
Introduction
The retainAll()
method in Java's ArrayList
class is a powerful tool for filtering list items based on specified conditions. It modifies the original ArrayList
by retaining only the elements that are also present in a specified collection, removing all other elements that do not meet the condition. This method is particularly useful when needing to extract a subset of elements that fulfill specific criteria from a larger group.
In this article, you will learn how to effectively use the retainAll()
method across various practical scenarios. Explore how this method helps in narrowing down lists to meet required conditions, with examples that demonstrate its application in different contexts.
Understanding the retainAll() Method
Basic Usage of retainAll()
Create two
ArrayList
instances, one containing the main list and the other containing elements to retain.Call the
retainAll()
method on the main list, passing the second list as an argument.javaArrayList<String> mainList = new ArrayList<>(Arrays.asList("Apple", "Banana", "Cherry", "Date")); ArrayList<String> retainList = new ArrayList<>(Arrays.asList("Banana", "Date")); mainList.retainAll(retainList); System.out.println(mainList);
This code retains only "Banana" and "Date" in
mainList
because these elements are also present inretainList
. The other elements are removed.
Managing Lists with Custom Objects
Define a custom class with proper
equals()
andhashCode()
implementations to ensure correct behavior ofretainAll()
.Create
ArrayList
instances of this custom class and useretainAll()
on them.javaclass Fruit { String name; int price; Fruit(String name, int price) { this.name = name; this.price = price; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; Fruit fruit = (Fruit) obj; return name.equals(fruit.name); } @Override public int hashCode() { return Objects.hash(name); } } ArrayList<Fruit> fruits = new ArrayList<>(); fruits.add(new Fruit("Apple", 100)); fruits.add(new Fruit("Banana", 30)); ArrayList<Fruit> retainFruits = new ArrayList<>(); retainFruits.add(new Fruit("Banana", 60)); fruits.retainAll(retainFruits); System.out.println(fruits.size()); // Output: 1
This example uses a custom
Fruit
class.retainAll()
checks the equality based on thename
field only, retaining the "Banana" object infruits
even though the prices differ.
Conclusion
The retainAll()
function in Java ArrayList
allows for effective filtering of lists based on specified conditions, significantly modifying the list to retain only desirable elements. Whether dealing with simple data types or custom objects, ensure relevant equality checks are in place to achieve the desired behavior. Use the retainAll()
method across various scenarios to refine your lists efficiently, leaving them with only the relevant data needed for further processing or analysis.
No comments yet.