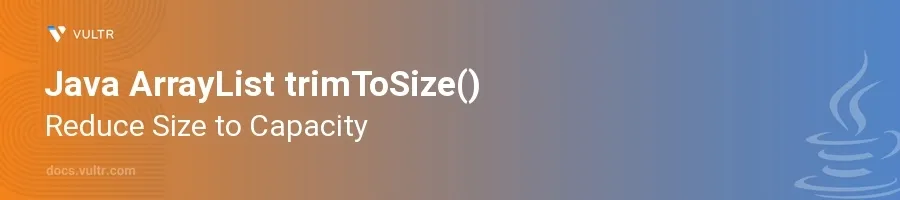
Introduction
The trimToSize()
method of the ArrayList
class in Java is a crucial tool for managing memory efficiently when working with dynamic arrays. This method adjusts the capacity of an ArrayList
instance to be equal to the list's current size. This operation is beneficial in scenarios where memory optimization is critical, such as in resource-constrained environments or when an ArrayList
will no longer be modified in size and its excess capacity needs reclaiming.
In this article, you will learn how to utilize the trimToSize()
method to optimize memory usage in your Java applications. Understand how this method works, its impact on the ArrayList
, and best practices for its use.
Understanding trimToSize()
What is trimToSize()?
- Realize that
ArrayList
in Java maintains an internal array to store its elements. - Recognize that the capacity of the
ArrayList
(the size of the internal array) can be larger than the actual number of elements it holds. - Use
trimToSize()
to reduce the storage capacity of theArrayList
to match the current number of elements, thus freeing up unused memory.
Why Use trimToSize()?
- Memory Efficiency: It helps in reducing the program's memory footprint by discarding unnecessary capacity.
- Performance Optimization: In certain contexts, it can improve performance by freeing up memory that might be used more effectively elsewhere.
Practical Usage of trimToSize()
Reducing ArrayList Capacity
Initialize an
ArrayList
with more capacity than required.Populate the
ArrayList
with elements.Apply
trimToSize()
to reduce the excess capacity.javaArrayList<Integer> numbers = new ArrayList<>(100); // Initial capacity of 100 numbers.add(1); numbers.add(2); numbers.add(3); numbers.trimToSize(); // Reduces the capacity to 3
This code snippet demonstrates creating an
ArrayList
with a capacity of 100, adding three elements, and then trimming the capacity down to the size of three elements usingtrimToSize()
.
Considering When to Use trimToSize()
- Consider using
trimToSize()
after completing the addition of elements if no more elements will be added. - Avoid using
trimToSize()
if theArrayList
is likely to grow again, as increasing the capacity later can be costly in terms of time and processing.
Conclusion
The trimToSize()
method in Java's ArrayList
class is a powerful tool for managing memory effectively. Its correct use ensures that you are only allocating as much memory as necessary, which can be critical in resource-constrained environments or to enhance overall application performance. By implementing the trimToSize()
method carefully and judiciously, you ensure that your Java applications remain efficient in their resource usage.
No comments yet.