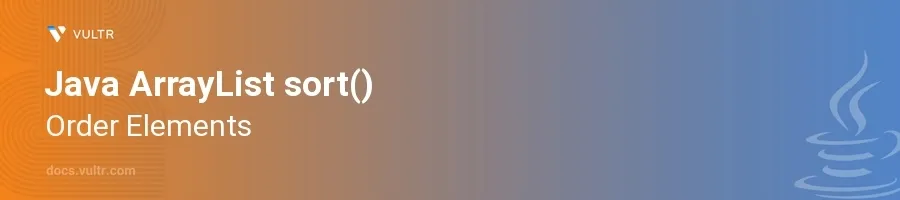
Introduction
The sort()
method in Java's ArrayList
class is a versatile tool for organizing elements in a specified order, whether ascending, descending, or by a custom-defined criteria. This method is crucial for data manipulation and is commonly applied in programs that need to process and present data systematically.
In this article, you will learn how to use the sort()
method effectively on ArrayList
objects in Java. Discover methods to sort elements and explore the versatility of the sort()
method by implementing it with various data types and custom sorting rules.
Using sort() with ArrayList
Sort in Natural Order
Instantiate an
ArrayList
and add elements to it.Use the
Collections.sort()
method to order the elements.javaimport java.util.ArrayList; import java.util.Collections; ArrayList<Integer> numbers = new ArrayList<>(); numbers.add(5); numbers.add(9); numbers.add(1); Collections.sort(numbers); System.out.println(numbers);
This code snippet initializes an
ArrayList
with integers, then sorts them in ascending order. The output will display the sorted list:[1, 5, 9]
.
Sort with Comparator
Understand that
Comparator
provides a way to define the custom order.Implement a comparator to sort the list in descending order.
Use the
Collections.sort()
with the comparator.javaimport java.util.ArrayList; import java.util.Collections; import java.util.Comparator; ArrayList<Integer> numbers = new ArrayList<>(); numbers.add(5); numbers.add(9); numbers.add(1); Collections.sort(numbers, Comparator.reverseOrder()); System.out.println(numbers);
Here,
Comparator.reverseOrder()
is used to sort the list in descending order. The result will show[9, 5, 1]
.
Custom Object Sorting
Define a class with multiple fields.
Create a comparator that defines a custom sort order based on one of the fields.
Sort an
ArrayList
of these objects using the custom comparator.javaimport java.util.ArrayList; import java.util.Collections; import java.util.Comparator; class Person { String name; int age; Person(String name, int age) { this.name = name; this.age = age; } @Override public String toString() { return this.name + " - " + this.age; } } ArrayList<Person> people = new ArrayList<>(); people.add(new Person("John", 30)); people.add(new Person("Alice", 24)); people.add(new Person("Bob", 27)); Collections.sort(people, new Comparator<Person>() { public int compare(Person p1, Person p2) { return Integer.compare(p1.age, p2.age); } }); System.out.println(people);
This example defines a
Person
class and sorts theArrayList
ofPerson
objects using a comparator based on the age attribute. The list will be sorted accordingly, displaying the people in ascending order of their ages.
Conclusion
The sort()
method in Java ArrayList
is essential for organizing elements, enhancing data manipulation, and improving data presentation. It is flexible, supporting natural ordering, reverse ordering, and highly customizable sorting through comparators. Familiarity with these sorting techniques elevates your capability to manage and process large datasets effectively, enforce order, and optimize data-related operations in Java applications. By mastering this method, ensure your Java programs are more structured and efficient.
No comments yet.