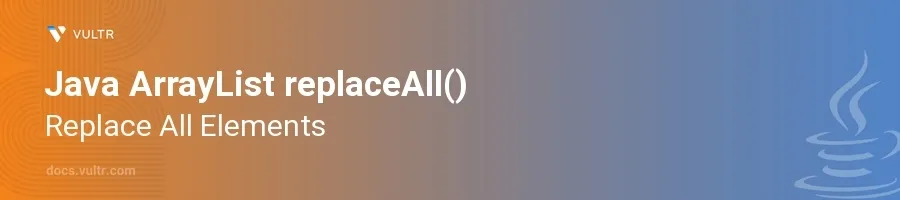
Introduction
The replaceAll()
method in Java's ArrayList
class provides a mechanism for modifying all elements of the list based on a specified rule or lambda expression. This allows for bulk transformations of elements, enhancing workflow efficiency when working with lists.
In this article, you will learn how to utilize the replaceAll()
method to modify ArrayList
elements in Java. This discussion will demonstrate practical uses of this method through various examples, illustrating how to apply lambda expressions to replace all elements according to certain conditions and rules.
Understanding replaceAll()
Basic Usage of replaceAll()
Create an
ArrayList
.Apply the
replaceAll()
method with a lambda expression that defines the new values of the elements based on a certain rule.javaArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5)); numbers.replaceAll(number -> number * 2); System.out.println(numbers);
This code doubles each element in the
ArrayList
. The lambda expressionnumber -> number * 2
serves as a rule, transforming each element by multiplying it by two.
Using Conditional Logic
Begin with an
ArrayList
.Use
replaceAll()
with a lambda expression incorporating conditional logic.javaArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5)); numbers.replaceAll(number -> (number % 2 == 0) ? number + 1 : number - 1); System.out.println(numbers);
In this example, the numbers are incremented by 1 if they are even and decremented by 1 if they are odd. The expression
(number % 2 == 0) ? number + 1 : number - 1
evaluates each element for this condition.
Advanced Usage
Integrating More Complex Transformations
Consider an
ArrayList
of objects.Replace each object based on more involved logic.
javaclass Person { String name; int age; Person(String name, int age) { this.name = name; this.age = age; } void birthday() { age++; } @Override public String toString() { return name + " is " + age; } } ArrayList<Person> persons = new ArrayList<>(); persons.add(new Person("Alice", 30)); persons.add(new Person("Bob", 25)); persons.replaceAll(person -> { person.birthday(); return person; }); System.out.println(persons);
Each
Person
object in the list has their age incremented through thebirthday()
method inside the lambda expression. This illustratesreplaceAll()
's capability to manipulate object properties in a convenient manner.
Conclusion
The replaceAll()
method in Java's ArrayList
class is a powerful tool for modifying list elements en masse using lambda expressions. It simplifies code meant for element transformation, whether the transformations are simple arithmetic operations, application of conditional logic, or more complex object manipulations. By mastering replaceAll()
, you enhance your capability to write clean, efficient, and effective Java code for manipulating collections.
No comments yet.