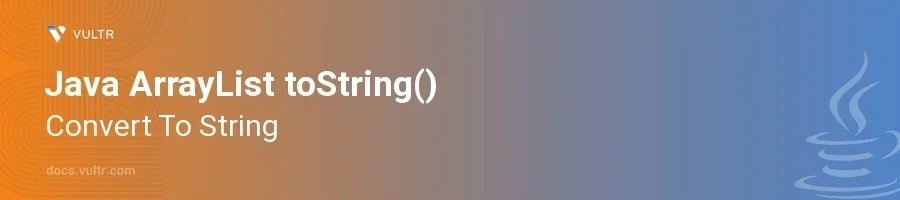
Introduction
The toString()
method in Java's ArrayList
class is a convenient way to convert the entire contents of the ArrayList into a single String. This feature proves useful when you need to quickly view or log the contents of an ArrayList or when you require a human-readable form of the list for output.
In this article, you will learn how to leverage the toString()
method to convert an ArrayList
into a string. Explore the default behavior of this method and how to customize it to suit specific formatting needs. Understand how this method can be used in practical scenarios to enhance data handling and representation in Java applications.
Understanding the Default toString() Behavior
Basic Conversion to String
Initialize an
ArrayList
.Add elements to the list.
Call the
toString()
method to convert the list into a string.javaimport java.util.ArrayList; ArrayList<String> colors = new ArrayList<>(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); String result = colors.toString(); System.out.println(result);
This code snippet prints
[Red, Green, Blue]
. ThetoString()
method converts theArrayList
into a string representation with elements enclosed in square brackets and separated by commas.
Displaying List of Custom Objects
Define a custom class with a properly overridden
toString()
method.Create an
ArrayList
of this custom class.Convert the
ArrayList
into a string usingtoString()
.javaimport java.util.ArrayList; class Product { private String name; private double price; public Product(String name, double price) { this.name = name; this.price = price; } @Override public String toString() { return String.format("%s: $%.2f", name, price); } } ArrayList<Product> products = new ArrayList<>(); products.add(new Product("Laptop", 999.99)); products.add(new Product("Smartphone", 499.99)); String result = products.toString(); System.out.println(result);
The overriding of the
toString()
method in theProduct
class enables a more detailed and formatted string output. This code outputs[Laptop: $999.99, Smartphone: $499.99]
.
Customizing toString() Output
Implementing a Custom List String Formatter
Create a method that iterates through the
ArrayList
and builds a custom string format.Use this method instead of the direct
toString()
call for more control.javaimport java.util.ArrayList; public static String customToString(ArrayList<?> list) { StringBuilder builder = new StringBuilder("["); for (int i = 0; i < list.size(); i++) { builder.append(list.get(i).toString()); if (i < list.size() - 1) { builder.append("; "); } } builder.append("]"); return builder.toString(); } ArrayList<Integer> numbers = new ArrayList<>(); numbers.add(10); numbers.add(20); numbers.add(30); String customResult = customToString(numbers); System.out.println(customResult);
Here, the custom
customToString
method formats the list elements separated by semicolons instead of commas. The output will be[10; 20; 30]
.
Conclusion
The toString()
method in Java's ArrayList
is instrumental for converting lists into readable string formats, enhancing both debugging and output capabilities. With it, embrace the simplicity of viewing entire lists in one go or adapt it through customization to match various formatting requirements. Grasp these concepts to enrich your data processing and ensure your Java applications communicate information effectively and clearly. By mastering the usage of the toString()
method in different contexts, enhance the robustness and readability of your code.
No comments yet.