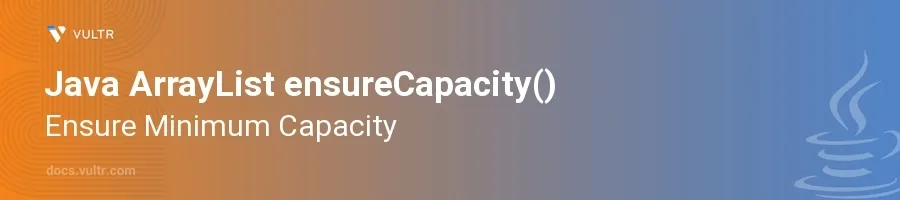
Introduction
The ensureCapacity()
method in Java's ArrayList
class is a critical tool for managing memory efficiently when working with dynamic arrays. This method helps optimize the array resizing process by ensuring that the array has the capacity to add a specified number of elements before needing to increase its size. This is particularly useful in scenarios where the size of the ArrayList is expected to grow substantially, thereby minimizing the need for frequent resizing.
In this article, you will learn how to effectively leverage the ensureCapacity()
method to manage array sizes proactively. Explore how to utilize this method to optimize memory and performance while working with ArrayLists in Java, alongside detailed examples demonstrating its usage and benefits.
Understanding ensureCapacity()
The ensureCapacity()
method is part of the ArrayList
class in Java's utility library. This method is used to increase the capacity of the ArrayList
if necessary, to ensure that it can hold at least the number of elements specified by the minimum capacity argument without needing to perform resizing operations.
When to Use ensureCapacity()
- Predict a significant increase in the size of an ArrayList.
- Minimize the overhead of resizing.
- Improve performance when large amounts of data are added to an ArrayList.
How ensureCapacity() Works
The ensureCapacity()
method helps by setting the internal capacity of an ArrayList to at least the number specified as a parameter. If the current capacity of the ArrayList is less than the argument, the list's capacity is increased. If the capacity is already equal or greater, the method does nothing.
The following example demonstrates how to use ensureCapacity()
:
Declare and initialize an ArrayList.
Use
ensureCapacity()
to set the desired capacity.javaimport java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> list = new ArrayList<>(); System.out.println("Initial Capacity: " + list.size()); // Ensure the capacity is at least 50 list.ensureCapacity(50); System.out.println("Capacity after ensuring: " + list.size()); } }
In this example, an
ArrayList
of Strings is created, andensureCapacity(50)
is called. This ensures that the ArrayList can store up to 50 elements before any reallocation of size is needed.
Use Cases and Benefits
Real-Time Data Handling
- Use
ensureCapacity()
when it's known in advance that a large dataset will be loaded into an ArrayList. - Prevent multiple resizings by setting a realistic initial capacity based on the data volume.
System Resource Management
- Utilize
ensureCapacity()
in environments with limited system resources. - Manage system memory more effectively by minimizing unnecessary memory allocation and garbage collection.
Efficiency in Batch Processes
- Batch operations that involve adding many elements to an ArrayList at once can benefit significantly from pre-adjusting the capacity.
- Reduce the computational cost of resizing the array multiple times during batch insertion processes.
Conclusion
The ensureCapacity()
method in Java's ArrayList class offers a way to proactively manage array capacity, which is crucial for optimizing performance and resource management in applications that deal with large data sets. By understanding when and how to use this method, you can significantly improve the efficiency of your Java applications. Always consider pre-setting capacities, especially in scenarios involving heavy data manipulation, to ensure smooth and efficient execution of your programs. Utilize the strategies discussed here to optimize your lists' capacity management effectively.
No comments yet.