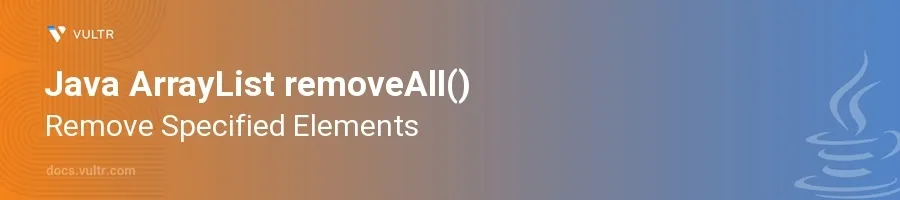
Introduction
The removeAll()
method in Java's ArrayList
class is a powerful tool for removing multiple elements from a list simultaneously based on the contents of another collection. This method simplifies tasks that require filtering out specific elements from a list, making it essential for data manipulation and cleanup operations in Java development.
In this article, you will learn how to effectively use the removeAll()
method in various scenarios. Explore how to leverage its capabilities to modify ArrayList
contents by removing elements that are specified in another collection. The practical examples provided will guide you through its usage and help you understand its behavior in different contexts.
Understanding removeAll()
The removeAll()
method is part of the Collection
interface and is implemented by the ArrayList
class among others. It removes all elements from the list that are also contained in the specified collection passed to the method.
Basic Usage of removeAll()
Create an
ArrayList
and populate it with elements.Create another
ArrayList
containing the elements to be removed.Use the
removeAll()
method to remove these elements from the first list.javaArrayList<String> originalList = new ArrayList<>(Arrays.asList("apple", "banana", "cherry", "date")); ArrayList<String> toRemoveList = new ArrayList<>(Arrays.asList("banana", "date")); originalList.removeAll(toRemoveList); System.out.println(originalList);
This code snippet creates two lists —
originalList
containing four fruit names andtoRemoveList
containing the names of the fruits to be removed. After callingremoveAll(toRemoveList)
, theoriginalList
will only contain "apple" and "cherry".
Considerations
- The elements in the
toRemoveList
need not be in the same order as inoriginalList
. - If an element in
toRemoveList
does not exist inoriginalList
, it has no effect. - The
removeAll()
method modifies the original list in place and returnstrue
if the list was changed.
Test for Successful Removal
Check the return value of the
removeAll()
method:javaboolean isModified = originalList.removeAll(toRemoveList); System.out.println("Was the original list modified? " + isModified);
The output will indicate whether elements were successfully removed from
originalList
.
Advanced Usage of removeAll()
Removing Objects That Meet Certain Conditions
Sometimes, you want to remove elements based on more complex conditions rather than just direct matches. Java 8 introduced Predicate
and stream features that can be used to handle such cases more flexibly.
Use a stream to filter and collect elements that should remain.
Replace the contents of the original list.
javaArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5, 6)); numbers.removeIf(n -> n % 2 == 0); // Remove all even numbers System.out.println(numbers);
This example removes all even numbers from the list. The
removeIf()
method is used here which takes aPredicate
as its argument for greater flexibility.
Conclusion
The removeAll()
method in Java's ArrayList
is a practical tool for efficiently removing specified elements from a list. Whether you're working with simple direct matches or need to apply more complex conditions, removeAll()
and related methods like removeIf()
significantly simplify list manipulation tasks. By integrating these techniques, you enhance your data handling capabilities in Java, making your code cleaner and more efficient.
No comments yet.