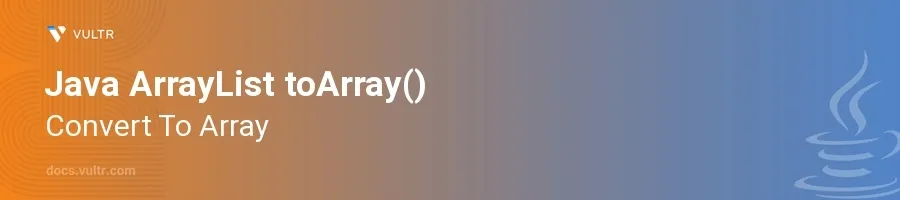
Introduction
The toArray()
method in Java's ArrayList
class is essential for converting an ArrayList to a standard array. This functionality is crucial when you need to pass the ArrayList data to APIs or libraries that only accept arrays or when performing operations that require array data structures.
In this article, you will learn how to use the toArray()
method in various scenarios, exploring both its basic form and its more advanced form that makes use of generics. Dive into practical code examples that demonstrate how to effectively convert an ArrayList into both Object arrays and specifically typed arrays.
Using toArray() to Convert to Object Array
Basic Conversion to an Object Array
Initialize an
ArrayList
.Add elements to the
ArrayList
.Use
toArray()
method to convert theArrayList
into anObject
array.javaArrayList<String> stringList = new ArrayList<>(); stringList.add("Apple"); stringList.add("Banana"); stringList.add("Cherry"); Object[] stringArray = stringList.toArray(); for(Object obj : stringArray) { System.out.println(obj); }
This code initializes an
ArrayList
of strings, adds a few elements, and then converts it to anObject[]
usingtoArray()
. The output will display each element.
Use in Conditional Statements
Convert an
ArrayList
directly within a conditional statement, such as a loop or if statement.Check if the array contains specific elements or meets a condition.
javaif (stringList.toArray().length > 0) { System.out.println("The list is not empty!"); }
Here,
toArray()
is used directly in anif
statement to check if the list is empty based on the array's length.
Using toArray(T[] a) with Typed Arrays
Specific Type Array Conversion
Create an
ArrayList
of a specific type.Convert the
ArrayList
to a typed array by passing a template array totoArray(T[] a)
.javaArrayList<String> colorList = new ArrayList<>(); colorList.add("Red"); colorList.add("Blue"); colorList.add("Green"); String[] colorArray = colorList.toArray(new String[0]); // Passing an empty array of String for(String color : colorArray) { System.out.println(color); }
This example demonstrates converting an
ArrayList<String>
to aString[]
. ThetoArray(new String[0])
ensures that the output array is of typeString
.
Handling Large Lists
Understand that providing an array of the appropriate size as the argument to
toArray(T[] a)
can increase efficiency.Ensure the array passed has the same or larger size than the
ArrayList
.javaString[] largeColorArray = colorList.toArray(new String[colorList.size()]);
The
toArray(new String[colorList.size()])
method here is used to ensure that the array is exactly the size of theArrayList
, which can be more memory-efficient for large lists.
Conclusion
Utilize the toArray()
method from Java's ArrayList
class to transition efficiently between collection-based and array-based data handling. Whether dealing with dynamic-sized collections or requiring a fixed-size array for API integrations, toArray()
provides the functionality needed to manage these scenarios seamlessly. By following the examples provided, simplify the process of converting collections into arrays in your Java applications, improving your code's adaptability and compatibility with various libraries and frameworks.
No comments yet.