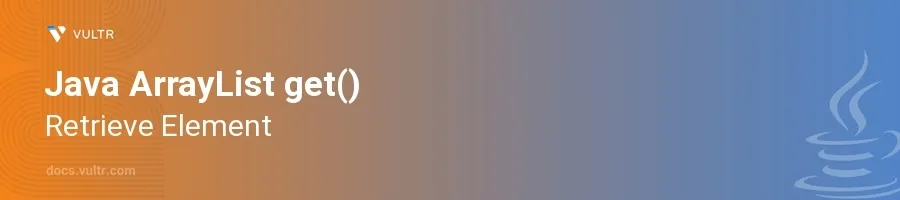
Introduction
The get()
method in Java's ArrayList
provides a simple yet powerful way to retrieve an element from a specified position in the list. This method is a part of the java.util.ArrayList
class, which implements the List
interface. It's commonly utilized in scenarios where you need to access an element by its index, making it crucial for data manipulation and retrieval in Java collections.
In this article, you will learn how to effectively use the get()
method in various programming scenarios. Understand how this method works, explore error handling associated with it, and review practical use cases to enhance your code robustness and readability.
Retrieving Elements with get()
Accessing a Single Element
Create an instance of
ArrayList
and add some elements.Use the
get()
method to retrieve an element by specifying its index.javaArrayList<String> colors = new ArrayList<>(); colors.add("Red"); colors.add("Blue"); colors.add("Green"); String element = colors.get(1); System.out.println(element);
This code snippet retrieves the element at index 1 (which is "Blue") from the
ArrayList
namedcolors
.
Handling IndexOutOfBoundsException
Implement error handling to manage scenarios where the index is out of the list’s range.
Use a
try-catch
block to catch theIndexOutOfBoundsException
.javatry { String element = colors.get(5); // Attempting to access an index out of bounds System.out.println(element); } catch (IndexOutOfBoundsException e) { System.out.println("The index is out of bounds: " + e.getMessage()); }
In this example, trying to access index 5, which does not exist in
colors
, triggers anIndexOutOfBoundsException
. The exception is caught and handled gracefully, displaying an error message.
Practical Use Cases of get()
Iterating Over Elements Using Index
Use a for loop with the
get()
method to iterate through all elements of anArrayList
.javafor (int i = 0; i < colors.size(); i++) { System.out.println(colors.get(i)); }
This approach allows access to each element in the
ArrayList
by its index, which can be useful for conditional processing inside the loop.
Retrieving Elements in a Specific Order
Access elements in reverse order using the
get()
method combined with a decremental loop.javafor (int i = colors.size() - 1; i >= 0; i--) { System.out.println(colors.get(i)); }
Accessing the list in reverse order is particularly useful in applications where the order of elements impacts the execution logic or output.
Conclusion
The get()
method in Java's ArrayList
is an essential tool for directly accessing elements by their index. Its straightforward implementation coupled with effective error handling makes it a reliable choice for developers. By adopting the get()
method in your applications, you enhance the versatility and functionality of your data handling capabilities. Whether iterating over elements, accessing them under conditional logic, or managing indices wisely to avoid exceptions, the get()
method provides both the simplicity and efficiency needed for effective programming.
No comments yet.