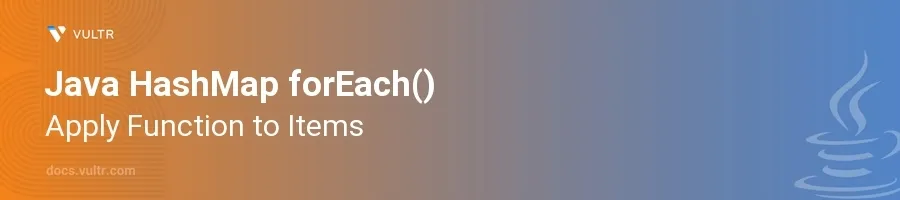
Introduction
The forEach()
method in Java’s HashMap class provides a way to iterate through each entry in the map and execute a specific action. This method, introduced in Java 8, simplifies iterating over map entries, enhances readability, and reduces the boilerplate code associated with traditional iteration techniques like using iterators or entry sets directly. It leverages functional programming features, particularly lambda expressions.
In this article, you will learn how to utilize the forEach()
method in various scenarios to apply functions to items in a Java HashMap. Discover how this method streamlines tasks such as printing values, modifying the contents of the map, and integrating more complex operations within your map data processing.
Working with forEach() in HashMaps
Print All Key-Value Pairs
Create a HashMap and add some key-value pairs.
Use the
forEach()
method to print each entry.javaHashMap<String, Integer> map = new HashMap<>(); map.put("One", 1); map.put("Two", 2); map.put("Three", 3); map.forEach((key, value) -> System.out.println(key + " = " + value));
This code initializes a
HashMap
with some integer values and prints out each key-value pair. The lambda expression specified inforEach()
takes two arguments (key
andvalue
) and performs theSystem.out.println()
action on each.
Modify Values Conditionally
Use
forEach()
to alter values based on a condition.Initialize a HashMap and adjust values depending on certain criteria.
javaHashMap<String, Integer> scoreMap = new HashMap<>(); scoreMap.put("Alice", 88); scoreMap.put("Bob", 55); scoreMap.put("Charlie", 92); scoreMap.forEach((name, score) -> { if (score < 60) { scoreMap.put(name, score + 20); // boosting scores less than 60 by 20 } });
Here, the code iterates over
scoreMap
and increases the score by 20 for students who originally scored less than 60. It's a straightforward way to apply conditions and modify values in a map.
Aggregate Map Values
Take advantage of
forEach()
to aggregate or summarize data from a HashMap.Start with a HashMap and compute a summary statistic.
javaHashMap<String, Integer> productStock = new HashMap<>(); productStock.put("Pens", 100); productStock.put("Pencils", 200); productStock.put("Markers", 50); final int[] totalStock = {0}; productStock.forEach((product, quantity) -> totalStock[0] += quantity); System.out.println("Total stock in store: " + totalStock[0]);
In this snippet, a sum of all stock quantities is calculated using
forEach()
. ThetotalStock
array is used to store the total because local variables referenced from within lambda expression should be final or effectively final.
Conclusion
The forEach()
method in Java’s HashMap class simplifies and optimizes the processing of entries in a map. By incorporating this method into your code, you streamline the iteration process, enhance readability, and make the code less error-prone. As demonstrated in various examples, whether it's printing data, modifying map contents, or calculating summaries, forEach()
proves to be a versatile tool for working with HashMap data efficiently. Embrace this method in various data handling scenarios to keep your Java code clean and expressive.
No comments yet.