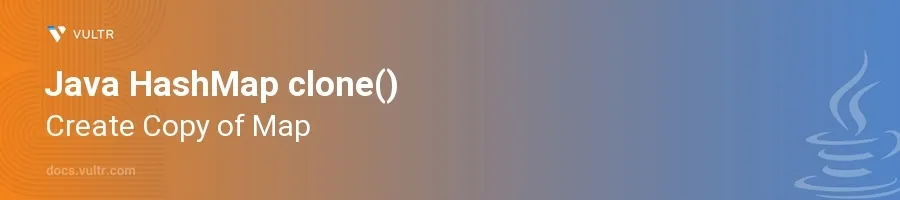
Introduction
The clone()
method in Java's HashMap
class provides a way to create a shallow copy of the existing map. This functionality is particularly useful when you want to maintain a separate copy of your map where modifications to the copied map do not affect the original map directly, although it's crucial to understand that this is a shallow copy, not a deep copy. This means only the structure of the map is copied, but the references to the objects remain the same.
In this article, you will learn how to leverage the clone()
method to copy HashMap
instances effectively. Explore how to implement cloning, recognize its limitations, and differentiate between shallow and deep copying.
Utilizing HashMap clone() Method
Basic HashMap Cloning
Create and populate a
HashMap
.Clone the
HashMap
using theclone()
method.javaimport java.util.HashMap; HashMap<Integer, String> originalMap = new HashMap<>(); originalMap.put(1, "One"); originalMap.put(2, "Two"); originalMap.put(3, "Three"); HashMap<Integer, String> clonedMap = (HashMap<Integer, String>) originalMap.clone(); System.out.println("Cloned Map: " + clonedMap);
This code snippet creates a
HashMap
, populates it with some key-value pairs, and then makes a shallow copy usingclone()
. PrintingclonedMap
will show the same contents asoriginalMap
.
Understanding Shallow Copy
Modify an entry in the cloned
HashMap
that links to a mutable object.Check the effect of this change on the original map.
javaHashMap<Integer, StringBuilder> originalMap = new HashMap<>(); originalMap.put(1, new StringBuilder("One")); originalMap.put(2, new StringBuilder("Two")); HashMap<Integer, StringBuilder> clonedMap = (HashMap<Integer, StringBuilder>) originalMap.clone(); // Modify the StringBuilder value in the cloned map clonedMap.get(1).append(" Modified"); System.out.println("Original Map: " + originalMap); System.out.println("Cloned Map: " + clonedMap);
Here, modifying the
StringBuilder
object inclonedMap
impacts the corresponding entry inoriginalMap
as well, showcasing the shallow nature ofclone()
.
Implementing a Deep Copy (Custom cloning)
Manually copy both keys and values if they are mutable.
javaHashMap<Integer, StringBuilder> deepCloneMap = new HashMap<>(); originalMap.forEach((key, value) -> deepCloneMap.put(new Integer(key), new StringBuilder(value.toString()))); // Now modify the StringBuilder in the cloned map deepCloneMap.get(1).append(" Deeply Modified"); System.out.println("Original Map: " + originalMap); System.out.println("Deep Clone Map: " + deepCloneMap);
This snippet manually creates a deep copy of
originalMap
by creating new instances of each key and value. Changes todeepCloneMap
do not affectoriginalMap
, demonstrating true independence.
Conclusion
The clone()
method in Java's HashMap
provides a convenient approach for creating a shallow copy of a map. While this method can be suitable for many scenarios, it's important to recognize the limitations of shallow copying, especially when working with mutable objects. For deep copying, manual implementation is necessary to avoid unintended data sharing. With this knowledge, confidently manage and manipulate copied HashMap
instances in Java according to your application's needs.
No comments yet.