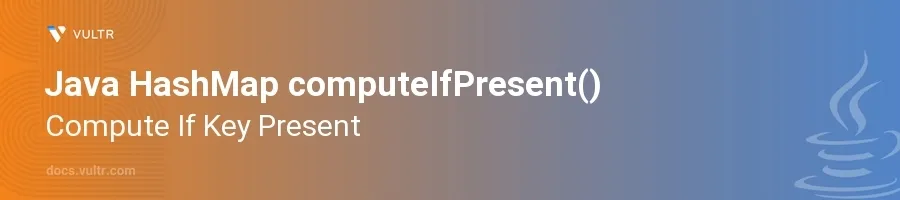
Introduction
The computeIfPresent()
method in Java's HashMap
class is a powerful tool for manipulating the value associated with a specific key if the key is already present in the map. This method is part of the Java Collections Framework and provides a way to compute the value of a mapping for specified keys using the given remapping function, allowing for more dynamic and efficient coding patterns.
In this article, you will learn how to effectively utilize the computeIfPresent()
method in various programming scenarios. Explore practical examples that demonstrate modifying map values conditionally, combining this method with lambda expressions for enhanced readability and functionality.
Understanding computeIfPresent() Basics
Syntax and Parameters
Familiarize yourself with the general syntax of the
computeIfPresent()
method:javaV computeIfPresent(K key, BiFunction<? super K, ? super V, ? extends V> remappingFunction)
Identify the key parameters:
key
: The key whose value needs to be computed.remappingFunction
: The function to compute a value given the key and its current mapped value.
Recognize the return value:
- Returns the new value associated with the specified key, or
null
if none.
- Returns the new value associated with the specified key, or
Basic Usage Example
Initialize a
HashMap
and apply thecomputeIfPresent()
method to modify an existing value.javaHashMap<String, Integer> map = new HashMap<>(); map.put("apple", 2); map.put("banana", 3); map.computeIfPresent("apple", (key, value) -> value + 5); System.out.println(map); // Outputs: {banana=3, apple=7}
In this example, the
computeIfPresent()
method increases the value associated with the key "apple" by 5. The lambda expression takes the original value and returns an increased value.
Advanced Usage Scenarios
Conditionally Modifying Values
Apply more complex conditions within the remapping function.
javaHashMap<String, Integer> stock = new HashMap<>(); stock.put("ankle boots", 12); stock.put("sneakers", 5); stock.computeIfPresent("ankle boots", (key, value) -> value > 10 ? value - 1 : value); System.out.println(stock); // Outputs: {sneakers=5, ankle boots=11}
Here, the stock of "ankle boots" is decreased by 1 only if the current stock is greater than 10. This illustrates conditional modification based on the existing value.
Combining with Other Operations
Leverage
computeIfPresent()
in conjunction with other map operations to create complex data manipulation flows.javaHashMap<String, String> userDetails = new HashMap<>(); userDetails.put("username", "john_doe"); userDetails.computeIfPresent("username", (key, value) -> value.toUpperCase()); userDetails.computeIfAbsent("email", k -> "john@example.com"); System.out.println(userDetails);
This demonstrates using
computeIfPresent()
to modify the username to uppercase. It also shows how to usecomputeIfAbsent()
to add a new entry if it doesn’t already exist in the map.
Conclusion
The computeIfPresent()
function in Java's HashMap
class offers an elegant way to conditionally modify the values of keys in a map based on complex logic. By integrating this method into your Java applications, you can achieve more maintainable and dynamic map operations, boosting both performance and readability. Employ the techniques discussed to refine your data management strategies in Java, ensuring that map manipulations are both efficient and intuitive.
No comments yet.