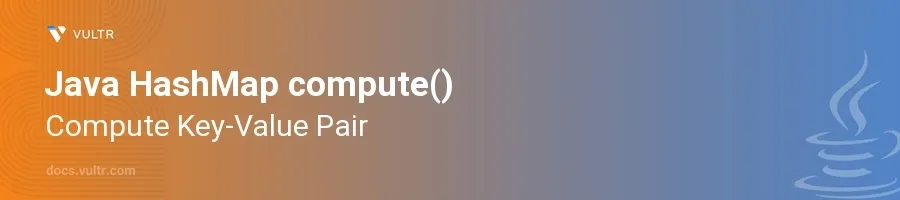
Introduction
The compute()
method in Java's HashMap
class allows for conditional processing and modification of key-value pairs directly within the map. This method is particularly useful for updating or inserting values based on specific conditions, enabling efficient in-place modifications without the need for separate retrieval, update, and put operations.
In this article, you will learn how to utilize the compute()
method to manipulate and manage key-value pairs in a HashMap
. Discover how this method optimizes data handling by applying functions conditionally, enhancing both readability and efficiency in Java applications.
Understanding the compute() Method
Basic Usage of compute()
Familiarize yourself with the
compute()
function signature: Thecompute()
method takes two parameters: a key and a remapping function. The remapping function specifies how the value for the specified key is computed.javaMap<String, Integer> map = new HashMap<>(); map.put("apple", 1); map.compute("apple", (key, value) -> value == null ? 1 : value + 1);
In this snippet, the
compute()
method checks if the current value of "apple" isnull
. If it is, it assigns1
; otherwise, it increments the existing value by1
.
Handling Absent Keys
Understand how
compute()
handles absent keys: If the key does not exist in the map, the second parameter of the remapping function will benull
. This is an opportunity to define how the map should handle such cases.javamap.compute("banana", (key, value) -> (value == null) ? 42 : value + 10);
Here, if "banana" does not exist in the map,
compute()
assigns it the value42
. If it exists, it increases the current value by10
.
Advanced Usage of compute()
Complex Value Calculation
Implement a more complex calculation or logic conditionally: The remapping function can contain any complex logic that may involve external or additional calculations.
javamap.compute("orange", (key, value) -> { if (value == null) return 10; int newVal = value * 10; return newVal > 100 ? 100 : newVal; });
This code snippet introduces a conditional cap on the value of "orange". If updating the value results in a value greater than
100
, it caps the value at100
.
Conclusion
Utilize the compute()
method in HashMap
to streamline the process of updating and managing key-value pairs directly. Embrace the flexibility it offers to implement complex conditional logic directly within the map structure, reducing the need for repetitive lookup and insert operations. Experiment with different scenarios and functions to fully leverage the capability of compute()
in maintaining and manipulating map data effectively.
No comments yet.