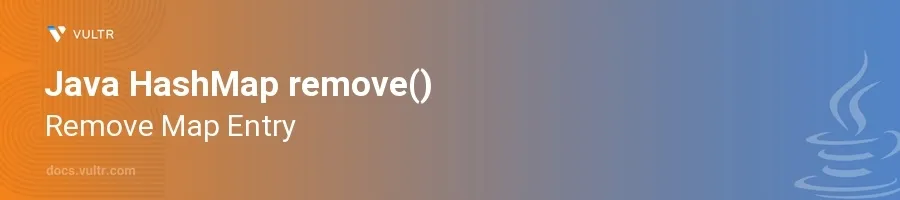
Introduction
The remove()
method in Java's HashMap
class is a crucial tool for managing entries in a map. It allows for the deletion of a specific entry based on its key, or optionally, based on both key and value. This functionality is essential when dynamically modifying the content of a map during runtime, such as when cleaning up resources or processing data entries.
In this article, you will learn how to efficiently use the remove()
method in various scenarios. Discover how to remove entries effectively from a HashMap
, handle special cases, and understand the method's impact on map performance.
Removing Entries Using Key
Remove an Entry by Key
Create a
HashMap
and populate it with some key-value pairs.Use the
remove()
method with the key of the entry that needs to be removed.javaHashMap<String, Integer> map = new HashMap<>(); map.put("One", 1); map.put("Two", 2); map.put("Three", 3); map.remove("Two"); System.out.println(map);
This snippet removes the entry with the key
"Two"
. After removal, the map contains only the entries for"One"
and"Three"
.
Check the Result of a Remove Operation
Capture the result of the
remove()
method to verify if an entry was actually removed.Use the returned value to perform further actions or checks.
javaHashMap<String, Integer> map = new HashMap<>(); map.put("A", 10); map.put("B", 20); Integer removedValue = map.remove("A"); System.out.println("Removed Value: " + removedValue);
In this scenario, the
remove()
method returns the value associated with the removed key, which is10
for the key"A"
. If the key does not exist,null
is returned.
Removing Entries Using Key and Value
Ensure Both Key and Value Match Before Removal
Use the
remove(Object key, Object value)
method to remove an entry only if both key and value match.This approach adds a layer of assurance that the correct entry is being removed, especially useful in cases with potential duplicate values or when precision is needed.
javaHashMap<String, Integer> map = new HashMap<>(); map.put("X", 30); map.put("Y", 45); map.put("Z", 30); boolean isRemoved = map.remove("Z", 30); System.out.println("Entry removed: " + isRemoved);
This code attempts to remove the entry where the key is
"Z"
and the value is30
. The method returnstrue
because the entry matches and is successfully removed. If either the key or value does not match, the method would returnfalse
, leaving the map unmodified.
Conclusion
The remove()
method in Java's HashMap
provides powerful capabilities for managing map entries, allowing easy removal of unnecessary or obsolete data. Understanding how to use both single-parameter and dual-parameter overloads of this method enhances the flexibility and reliability of your code. By leveraging these techniques, you maintain cleaner and more efficient data structures in your Java applications.
No comments yet.