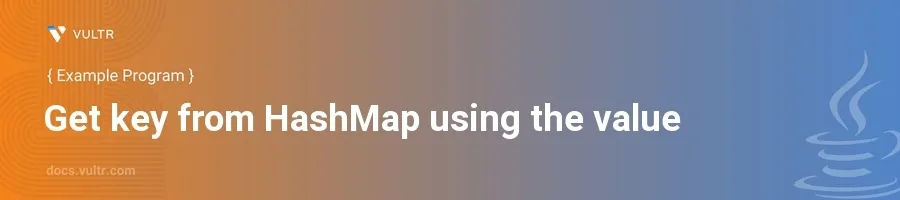
Introduction
Java's HashMap
class simplifies the storage and retrieval of data with its efficient mapping of keys to values. Typically, one accesses the value by knowing the key. However, situations may arise where you need to determine the key associated with a specific value. This can be particularly useful in applications where relationships or mappings might be evaluated or inverted for specific logic operations.
In this article, you will learn how to retrieve a key from a HashMap
in Java using its value. Explore several methods and examples that outline different scenarios and provide you with clear guidelines on handling such cases effectively using Java programming constructs.
Finding Key by Value in HashMap
Basic Method: Iteration Over EntrySet
Understand that
HashMap
does not have a direct method to find keys by values because the mapping is designed to be accessed by keys.Create a method that iterates over the entry set of the
HashMap
to find the key for a specific value.javaimport java.util.HashMap; import java.util.Map; public class KeyFinder { public static <K, V> K getKey(HashMap<K, V> map, V value) { for (Map.Entry<K, V> entry : map.entrySet()) { if (entry.getValue().equals(value)) { return entry.getKey(); } } return null; } }
This method,
getKey
, accepts aHashMap
and the value you are looking for. It iterates through each entry in the map, checking if the entry's value matches the specified value. If a match is found, the corresponding key is returned.
Handling Multiple Keys for a Value
Modify the approach to handle scenarios where multiple keys might map to the same value.
Instead of returning a single key, return a list of keys that match the value.
javaimport java.util.HashMap; import java.util.Map; import java.util.ArrayList; import java.util.List; public class KeyFinder { public static <K, V> List<K> getKeys(HashMap<K, V> map, V value) { List<K> keys = new ArrayList<>(); for (Map.Entry<K, V> entry : map.entrySet()) { if (entry.getValue().equals(value)) { keys.add(entry.getKey()); } } return keys; } }
In this revised version,
getKeys
returns aList
of keys. It collects all keys that correspond to the specified value and adds them to anArrayList
. This method is useful when duplicates of the value exist within the map.
Example Execution
Demonstrate the working of these methods with a practical example.
Create a
HashMap
and populate it with data.Retrieve keys using the methods defined above.
javapublic class Main { public static void main(String[] args) { HashMap<String, Integer> map = new HashMap<>(); map.put("Blue", 1); map.put("Red", 2); map.put("Green", 2); map.put("Yellow", 3); String key = KeyFinder.getKey(map, 2); System.out.println("Key for Value 2: " + key); List<String> keys = KeyFinder.getKeys(map, 2); System.out.println("Keys for Value 2: " + keys); } }
This example initializes a
HashMap
with colors as keys and integers as values. It then retrieves a key for the value2
using both methods. ThegetKey
method returns the first key it finds, while thegetKeys
method returns all keys associated with the value2
.
Conclusion
Retrieving a key from a HashMap
using a value requires iterating through the map since HashMap
is designed primarily for key-based access. The examples provided in this article help you understand how to implement these methodologies in Java applications effectively. Whether you’re dealing with unique or non-unique values, these strategies ensure you can reverse-lookup keys from values in any given map scenario. Adapt these methods to fit your specific requirements, enhancing the functionality and versatility of your Java programs.
No comments yet.