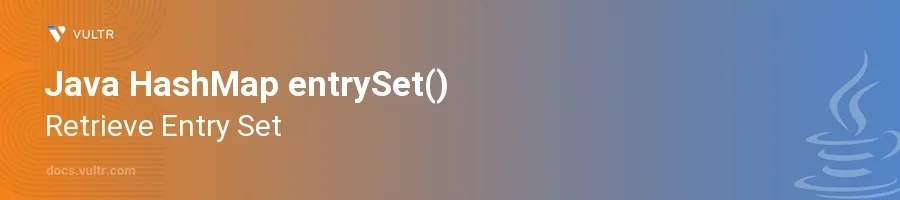
Introduction
The entrySet()
method in Java's HashMap
is an essential tool for accessing the key-value pairs within the map. This method returns a set view of the mappings contained in the map, enabling you to interact with its contents via set operations like iteration. Understanding how to use the entrySet()
effectively can enhance the way you manipulate data structures in Java.
In this article, you will learn how to retrieve and manipulate the entry set from a Java HashMap
. Discover the various scenarios where entrySet()
proves valuable, and explore techniques to iterate over these sets and perform operations on the key-value pairs.
Retrieving Entry Set from HashMap
Basic Usage of entrySet()
Declare and initialize a
HashMap
.Use the
entrySet()
method to get the set view of the mappings.javaHashMap<String, Integer> map = new HashMap<>(); map.put("apple", 10); map.put("banana", 5); map.put("cherry", 20); Set<Map.Entry<String, Integer>> entries = map.entrySet();
This code snippet creates a
HashMap
with some fruit names and their corresponding quantities. It then retrieves the set view of this map usingentrySet()
, allowing further manipulation or iteration.
Iterating Over the Entry Set
Retrieve the entry set from a
HashMap
as shown in the previous steps.Use a for-each loop to iterate over the set.
javafor (Map.Entry<String, Integer> entry : entries) { System.out.println(entry.getKey() + " has " + entry.getValue() + " items"); }
In this snippet, each entry in the
HashMap
is printed out. ThegetKey()
andgetValue()
methods allow access to the key and value of each entry respectively.
Modifying Values During Iteration
Iterate over the entry set of a
HashMap
using a for-each loop.Modify the value of an entry during iteration.
javafor (Map.Entry<String, Integer> entry : entries) { entry.setValue(entry.getValue() * 2); // Double the quantity }
This example doubles the quantity of each item in the map during the iteration process. Modifying the contents via the entry set view reflects changes directly in the original
HashMap
.
Conclusion
The entrySet()
method of the HashMap
class in Java is a dynamic way to interact with the map's contents, allowing not only read access but also modifications to the elements. By utilizing the entry set, you optimize your data handling capabilities in Java, making your code more efficient and readable. Implement the outlined methods in various use cases to maximize the potential of your data structures and ensure robust management of key-value pairs in your programs.
No comments yet.