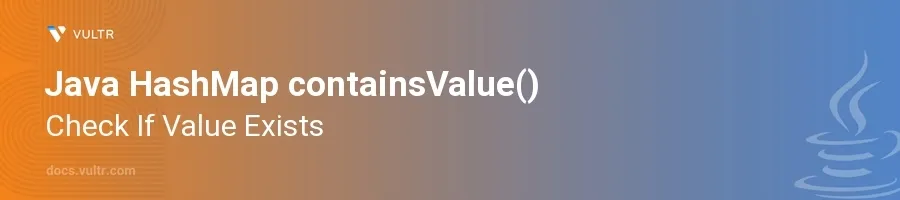
Introduction
The containsValue()
method in Java's HashMap class serves as an essential tool for determining whether a specific value exists within the map. This method provides a straightforward approach for checking presence, which aids significantly in decision-making processes in your applications.
In this article, you will learn how to effectively utilize the containsValue()
method with HashMaps. Explore practical examples that demonstrate checking for the existence of various values, including objects and custom data types.
Using containsValue() with Primitive and Wrapper Types
Check for the Presence of a Specific Integer
Create a HashMap and populate it with integer key-value pairs.
Use the
containsValue()
method to determine if a specific integer value is in the map.javaHashMap<Integer, Integer> map = new HashMap<>(); map.put(1, 100); map.put(2, 200); map.put(3, 300); boolean exists = map.containsValue(200); System.out.println("Value 200 exists: " + exists);
This code initializes a HashMap with integer values and checks if the value
200
is present. The output will indicate whether the value is found.
Searching for a String Value
Start by setting up a HashMap with string types.
Apply
containsValue()
to check for a specific string in the values.javaHashMap<Integer, String> nameMap = new HashMap<>(); nameMap.put(1, "Alice"); nameMap.put(2, "Bob"); boolean found = nameMap.containsValue("Alice"); System.out.println("Value 'Alice' found: " + found);
Here, the HashMap holds names as values, and the code searches for the presence of "Alice". The result will reflect if the name "Alice" is part of the HashMap values.
Working with Custom Objects
Check for Object Presence Using containsValue()
Define a custom class and override the
equals()
method for accurate comparison.Initialize a HashMap to hold your custom objects.
Insert values into the map and use
containsValue()
to find a specific object.javaclass Person { String name; int age; Person(String name, int age) { this.name = name; this.age = age; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; Person person = (Person) obj; return age == person.age && Objects.equals(name, person.name); } } HashMap<Integer, Person> peopleMap = new HashMap<>(); Person alice = new Person("Alice", 30); peopleMap.put(1, alice); Person searchPerson = new Person("Alice", 30); boolean personExists = peopleMap.containsValue(searchPerson); System.out.println("Person exists: " + personExists);
This snippet demonstrates checking for a specific
Person
object in the HashMap. Theequals()
method ensures that twoPerson
objects are considered equal if they have the same name and age.
Conclusion
The containsValue()
method in Java's HashMap is a fundamental tool for checking if a specific value exists within the map, offering versatility in handling not only primitive and wrapper types but also custom objects. Apply this method to unify code logic, maintain clarity, and improve decision-making tasks in your software development. Through the strategies discussed, you master efficient value-checking techniques that enhance the functionality and manageability of Java applications.
No comments yet.