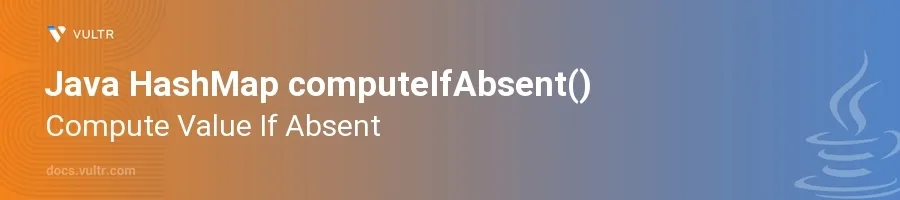
Introduction
The computeIfAbsent()
method in Java's HashMap
class is a powerful tool for optimizing data storage and retrieval operations. This method helps by computing a value for a specified key if it is not already associated with a value, or it is mapped to null
. It is particularly useful in scenarios where defaulting values and avoiding unnecessary computations or overwrites are critical.
In this article, you will learn how to leverage the computeIfAbsent()
method effectively in Java HashMaps. Explore practical use cases where this method can streamline your code while maintaining efficiency when dealing with a map’s keys and values.
Understanding computeIfAbsent()
Basic Usage
Grasp the purpose of
computeIfAbsent()
.Utilize the method to insert a default value if the key is absent or null in the map.
javaimport java.util.HashMap; import java.util.Map; Map<String, Integer> map = new HashMap<>(); Integer value = map.computeIfAbsent("key1", k -> 5); System.out.println("Value for 'key1': " + value);
In this example,
computeIfAbsent()
checks if "key1" is absent in the mapmap
. Since it is, it computes the value using the lambda expressionk -> 5
, resulting inkey1
being associated with5
.
Lazy Computation Benefits
Understand the lazy computing aspect of
computeIfAbsent()
.Apply the method where a value should be computed and stored only when needed.
javaimport java.util.HashMap; import java.util.Map; Map<String, String> usernameMap = new HashMap<>(); String username = "user123"; String result = usernameMap.computeIfAbsent(username, k -> getUsernameFromDatabase(k));
In this code, the username "user123" might not exist in
usernameMap
. Instead of preemptively loading data from a database,computeIfAbsent()
fetches and inserts the data only ifuser123
is not already a key. This prevents unnecessary database calls.
Application in Complex Data Structures
Realize the adaptability of
computeIfAbsent()
in managing more intricate data structures.Employ the method to automate and simplify management of nested maps.
javaimport java.util.HashMap; import java.util.Map; Map<String, Map<Integer, String>> userLogs = new HashMap<>(); userLogs.computeIfAbsent("userID", k -> new HashMap<>()).put(123, "Logged in");
Here, the method manages a map of maps. If the map for a certain
userID
doesn't exist, it initializes a new one and directly logs an event.
Advanced Tips
Handling null Values
- Remember that
computeIfAbsent()
will not compute a value if the key is explicitly associated withnull
. Consider usingcompute()
if dealing with such cases.
Performance Considerations
- Optimize performance by ensuring that the lambda expression or method reference used in
computeIfAbsent()
is efficient, especially when handling large sets of data.
ConcurrentHashMap Compatibility
- Note that
computeIfAbsent()
works similarly inConcurrentHashMap
, making it suitable for thread-safe operations.
Conclusion
The computeIfAbsent()
method in Java's HashMap
optimizes handling of absence conditions in maps by computing and inserting values only when necessary. This functionality not only cleans up code by reducing redundancy but also enhances performance by preventing unnecessary computations. Use it to maintain clean, readable, and efficient code, especially when working with complex data structures or managing default values dynamically.
No comments yet.