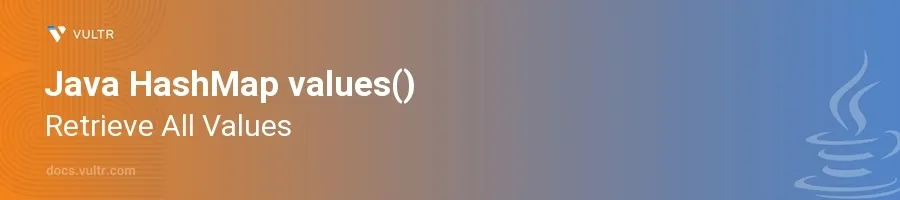
Introduction
The values()
method in Java's HashMap
class provides a straightforward way to access all the values stored in a map. This method is part of the Java Collections Framework and returns a collection view of the values contained in the map. Understanding how to effectively use this method can significantly enhance your ability to manipulate and process sets of data within your applications.
In this article, you will learn how to effectively retrieve all values from a HashMap
using the values()
method. Explore practical examples that demonstrate its usage in various scenarios and see how it integrates into larger programming tasks.
Retrieving Values with values()
Basic Usage of values()
Start by creating a
HashMap
.Populate the
HashMap
with some key-value pairs.Use the
values()
method to fetch the values.javaimport java.util.HashMap; import java.util.Collection; public class Main { public static void main(String[] args) { HashMap<Integer, String> map = new HashMap<>(); map.put(1, "Java"); map.put(2, "Python"); map.put(3, "C++"); Collection<String> values = map.values(); System.out.println("Values: " + values); } }
This code initializes a
HashMap
with keys of typeInteger
and values of typeString
. It then retrieves the values usingvalues()
and prints them. The output would be a collection of values:Values: [Java, Python, C++]
.
Analysing the Returned Collection
Recognize that the collection returned by
values()
is a view.Understand that modifications to this collection reflect in the original map and vice versa.
javaHashMap<Integer, String> map = new HashMap<>(); map.put(1, "Java"); Collection<String> values = map.values(); values.remove("Java"); // Reflects on the original map System.out.println("Updated Map: " + map);
Removing an item from the
values
collection also removes the corresponding mapping from theHashMap
. The updatedmap
would now be{2=Python, 3=C++}
after the removal.
Use Case: Processing Values
Use the retrieved collection to perform operations such as filtering or aggregation.
Apply Java Stream API for more complex operations.
javaimport java.util.HashMap; import java.util.stream.Collectors; public class Example { public static void main(String[] args) { HashMap<Integer, String> map = new HashMap<>(); map.put(1, "Apple"); map.put(2, "Banana"); map.put(3, "Carrot"); map.put(4, "Date"); // Filter values that start with a consonant java.util.List<String> filteredValues = map.values().stream() .filter(value -> !value.matches("^[AEIOUaeiou].*")) .collect(Collectors.toList()); System.out.println("Filtered Values: " + filteredValues); } }
This snippet demonstrates using streams to filter values in the
HashMap
that start with consonants. The result would beFiltered Values: [Banana, Carrot, Date]
.
Conclusion
Using the values()
method in a Java HashMap
provides a convenient way to access all values stored in the map. This method returns a view of the map's values, allowing for dynamic changes reflected across the map and the view. Whether simply retrieving these values for display or manipulating them through more complex operations like filtering or aggregation, understanding how to utilize the values()
method enhances your Java programming skills. Equip yourself with these techniques to manage and process large sets of data efficiently. By applying these skills, you ensure your Java applications are robust and effective at handling data-centric operations.
No comments yet.