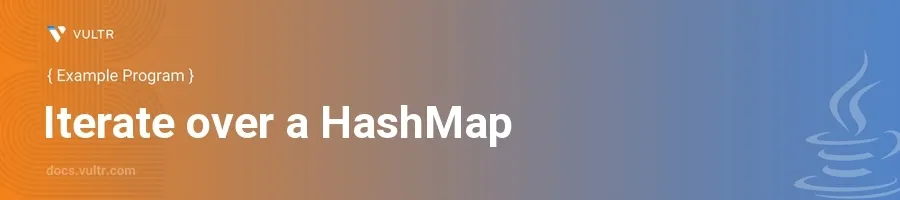
Introduction
Iterating over a HashMap
in Java is a common task that you might encounter while developing Java applications. A HashMap
stores items in "key/value" pairs and allows for fast retrieval based on keys. Understanding how to properly iterate through a HashMap
is essential for tasks such as displaying its content, modifying values, or performing aggregate computations.
In this article, you will learn how to efficiently iterate over a HashMap
using several methods available in Java. Utilize for loops, iterator objects, and lambda expressions to explore different ways to traverse and interact with the elements in your HashMap
.
Basic Iteration Techniques
Using a for-each Loop to Access Keys and Values
Start by initializing a
HashMap
and populating it with some data.Use a for-each loop to iterate through the key set of the
HashMap
.javaimport java.util.HashMap; HashMap<String, Integer> map = new HashMap<>(); map.put("Alice", 23); map.put("Bob", 27); map.put("Charlie", 31); for (String key : map.keySet()) { Integer value = map.get(key); System.out.println(key + " : " + value); }
This code initializes a
HashMap
with some names and ages, then iterates through the keys to retrieve and print each corresponding value.
Iterating Over Entry Set with a for-each Loop
Use the
entrySet()
method to get a set view of the mappings contained in theHashMap
.Iterate over each entry (key-value pair) in the set.
javafor (HashMap.Entry<String, Integer> entry : map.entrySet()) { System.out.println(entry.getKey() + " : " + entry.getValue()); }
This snippet uses the
entrySet()
method, which allows direct access to both keys and values at the same time, thus improving the efficiency of the iteration.
Advanced Iteration Methods
Using an Iterator
Obtain an iterator from the
entrySet()
of theHashMap
.Loop through the
HashMap
using theIterator
object.javaimport java.util.Iterator; import java.util.Map; Iterator<Map.Entry<String, Integer>> iterator = map.entrySet().iterator(); while (iterator.hasNext()) { Map.Entry<String, Integer> entry = iterator.next(); System.out.println(entry.getKey() + " : " + entry.getValue()); }
This code snippet demonstrates the use of an
Iterator
to loop through aHashMap
. It is particularly useful when you need to remove entries during iteration without causing aConcurrentModificationException
.
Using Lambda Expressions in Java 8 and Beyond
Take advantage of Java 8's lambda expressions to succinctly iterate over a
HashMap
.Use the
forEach
method introduced in Java 8.javamap.forEach((key, value) -> System.out.println(key + " : " + value));
Lambda expressions provide a clear and concise way to iterate over
HashMap
entries. TheforEach
method allows applying an action to each entry of the map, streamlining the code for readability and efficiency.
Functional Programming Techniques
Stream API with Filters
Use the Java
Stream
API to process entries based on certain conditions.Filter entries and perform an action on the filtered set.
javamap.entrySet().stream() .filter(e -> e.getValue() > 25) .forEach(e -> System.out.println(e.getKey() + " : " + e.getValue()));
This example filters the
HashMap
to only include entries where the age is greater than 25, then prints each of those entries. Working withStream
allows for complex manipulations of collections using a functional approach.
Conclusion
Iterating over a HashMap
in Java can be performed in various ways, each suitable for different scenarios and optimization needs. From basic loops to advanced streams and lambda expressions, select the method that best fits your data operations and performance objectives. Ensuring proficiency with these techniques enhances coding efficiency and fluency in managing key-value pairs within your Java applications. Adapt these iteration strategies to explore and manipulate HashMap
contents effectively.
No comments yet.